Change the style of a modal using react-modal
Solution 1
It should be portalClassName:
<Modal
isOpen={this.state.modalIsOpen}
onRequestClose={this.closeModal}
portalClassName="modal"
>
Solution 2
I think there might be a billion ways to do this, here is just one that uses CSS Modules. If you put your styles into a separate .css.js file you can import it in your module:
/// modal.css.js ///
export default {
modal: {
top: '35%',
left: '50%',
right: 'auto',
bottom: 'auto',
marginRight: '-50%',
width: '60%',
transform: 'translate(-40%, -10%)'
},
greenText: {
color: 'rgb(0,255,100)'
},
style3: {
marginRight: '-25%'
}
}
You can then assign your styles by accessing them as you would with any object and apply them to your component on the style attribute
import styles from './modal.css.js'
...
<Modal
isOpen={this.state.modalIsOpen}
onRequestClose={this.closeModal}
style={styles.modal}
>
if you want to apply multiple styles to your component you give the style attribute an array. This would allow you to apply styles from multiple imported style objects.
<Modal
isOpen={this.state.modalIsOpen}
onRequestClose={this.closeModal}
style={[styles.modal, styles.greenText]}
>
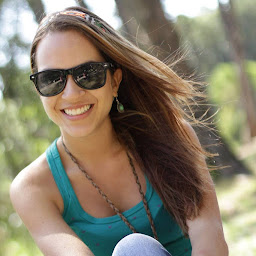
Comments
-
Liz Parody almost 2 years
I have this object, with the styles I want for the modal:
const customStyles = { content: { top: '35%', left: '50%', right: 'auto', bottom: 'auto', marginRight: '-50%', width: '60%', transform: 'translate(-40%, -10%)', }, };
Then I pass that styles to the modal like this:
<Modal isOpen={this.state.modalIsOpen} onRequestClose={this.closeModal} style={customStyles} >
And it works fine but I want to pass a class, not create a customStyle object inside the component.
I try something like this, first creating the modal class:
.modal { top: '35%'; left: '50%'; right: 'auto'; bottom: 'auto'; marginRight: '-50%'; width: '60%'; transform: 'translate(-40%, -10%)'; }
and then:
<Modal isOpen={this.state.modalIsOpen} onRequestClose={this.closeModal} className="modal" >
But it didn't work. What am I doing wrong?
-
JimmyM over 5 yearsThis completely solves OP's (and my) issue and should really be the accepted answer imo.
-
haz over 4 yearsHow can you style the background color of the modal (i.e. the overlay) this way?
-
johnjohn over 3 years@haz you would follow the convention of an
.overlay { }
class, then add this to the Modal props:overlayClassName={styles.overlay}
-
helvete over 2 yearsThank you for this code snippet, which might provide some limited, immediate help. A proper explanation would greatly improve its long-term value by showing why this is a good solution to the problem and would make it more useful to future readers with other, similar questions. Please edit your answer to add some explanation, including the assumptions you’ve made.