Convert a file path to Uri in Android
Solution 1
Please try the following code
Uri.fromFile(new File("/sdcard/sample.jpg"))
Solution 2
Normal answer for this question if you really want to get something like content//media/external/video/media/18576
(e.g. for your video mp4 absolute path) and not just file///storage/emulated/0/DCIM/Camera/20141219_133139.mp4
:
MediaScannerConnection.scanFile(this,
new String[] { file.getAbsolutePath() }, null,
new MediaScannerConnection.OnScanCompletedListener() {
public void onScanCompleted(String path, Uri uri) {
Log.i("onScanCompleted", uri.getPath());
}
});
Accepted answer is wrong (cause it will not return content//media/external/video/media/*
)
Uri.fromFile(file).toString()
only returns something like file///storage/emulated/0/*
which is a simple absolute path of a file on the sdcard but with file//
prefix (scheme)
You can also get content
uri using MediaStore
database of Android
TEST (what returns Uri.fromFile
and what returns MediaScannerConnection
):
File videoFile = new File("/storage/emulated/0/video.mp4");
Log.i(TAG, Uri.fromFile(videoFile).toString());
MediaScannerConnection.scanFile(this, new String[] { videoFile.getAbsolutePath() }, null,
(path, uri) -> Log.i(TAG, uri.toString()));
Output:
I/Test: file:///storage/emulated/0/video.mp4
I/Test: content://media/external/video/media/268927
Solution 3
If you want to Get Uri path from String File path .this code will be worked also in androidQ.
String outputFile = context.getExternalFilesDir("DirName") + "/fileName.extension";
File file = new File(outputFile);
Log.e("OutPutFile",outputFile);
Uri uri = FileProvider.getUriForFile(Activity.this,
BuildConfig.APPLICATION_ID + ".provider",file);
declare provider in application Tag manifest
<provider
android:name="androidx.core.content.FileProvider"
android:authorities="${applicationId}.provider"
android:exported="false"
android:grantUriPermissions="true">
<meta-data
android:name="android.support.FILE_PROVIDER_PATHS"
android:resource="@xml/provider_paths" />
</provider>
under res -> xml ->provider_paths.xml
<paths>
<external-path name="external_files" path="."/>
</paths>
Related videos on Youtube
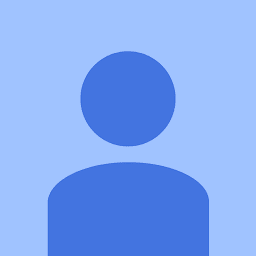
Vinodh Kumar
Updated on March 03, 2021Comments
-
Vinodh Kumar about 3 years
I have an app where I capture a video using the camera. I can get the video's file path, but I need it as a Uri.
The file path I'm getting:
/storage/emulated/0/DCIM/Camera/20141219_133139.mp4
What I need is like this:
content//media/external/video/media/18576.
This is my code.
protected void onActivityResult(int requestCode, int resultCode, Intent data) { // if the result is capturing Image if (requestCode == CAMERA_CAPTURE_VIDEO_REQUEST_CODE) { if (resultCode == RESULT_OK) { // video successfully recorded // preview the recorded video // selectedImageUri = data.getData(); // Uri selectedImage = data.getData(); previewVideo(); tv1.setText(String.valueOf((fileUri.getPath()))); String bedroom=String.valueOf((fileUri.getPath())); Intent i = new Intent(); i.putExtra(bhk1.BEDROOM2, bedroom); setResult(RESULT_OK,i); btnRecordVideo.setText("ReTake Video"); } else if (resultCode == RESULT_CANCELED) { // user cancelled recording Toast.makeText(getApplicationContext(), "User cancelled video recording", Toast.LENGTH_SHORT) .show(); } else { // failed to record video Toast.makeText(getApplicationContext(), "Sorry! Failed to record video", Toast.LENGTH_SHORT) .show(); } } }
I need a Uri from the String variable
bedroom
.-
Rajesh Mikkilineni over 9 yearspossible duplicate of Get content uri from file path in android
-
greenapps over 9 years
// Uri selectedImage = data.getData();
. Well .. isn't that your uri? -
greenapps over 9 years
fileUri.getPath()
. What is filePath? You should have told/shown! -
greenapps over 9 years
I am getting the path is /storage/emulated/0/DCIM/Camera/20141219_133139.mp4
. Where? We have to guess? -
Vinodh Kumar over 9 yearsafter i record the video the storage path path is /storage/emulated/0/DCIM/Camera/20141219_133139.mp4.i need to get like this content//media/external/video/media/18576
-
greenapps over 9 yearsPlease react on my comments instead of repeating your question. I understood your question already.
-
Vinodh Kumar over 9 yearsI want to upload the video to youtube for that the path string is not working,so that i need the video file uri like this content//media/external/video/media/18576
-
GvSharma over 8 yearsHi vinod...did you solve this? i need image uri to upload it to server.
-
-
10101010 about 5 yearscould be helpful to take a look here: stackoverflow.com/questions/38200282/…
-
grrigore almost 4 yearsYou can use
file.toUri()
for Kotlin -
KMC almost 4 years@grrigore I think you meant toURI() which belongs to java.net package.
-
grrigore almost 4 years@KMC developer.android.com/reference/kotlin/androidx/core/net/… this is the one I'm talking about
-
aryanknp over 3 yearswhats the difference between
content//
andfile///
, I ask because both works in my case -
Minimus Heximus over 3 yearsplease provide a function that gets path and returns uri.
-
user924 over 2 years@aryanknp read about MediaStore
-
Ramesh about 2 yearsThis answer is the only working answer. Above accepted answer doesn't solve the problem.