Converting an image to base64 in angular 2
Solution 1
Working plunkr for base64 String
https://plnkr.co/edit/PFfebmnqH0eQR9I92v0G?p=preview
handleFileSelect(evt){
var files = evt.target.files;
var file = files[0];
if (files && file) {
var reader = new FileReader();
reader.onload =this._handleReaderLoaded.bind(this);
reader.readAsBinaryString(file);
}
}
_handleReaderLoaded(readerEvt) {
var binaryString = readerEvt.target.result;
this.base64textString= btoa(binaryString);
console.log(btoa(binaryString));
}
Solution 2
I modified Parth Ghiya answer a bit, so you can upload 1- many images, and they are all stored in an array as base64 encoded strings
base64textString = [];
onUploadChange(evt: any) {
const file = evt.target.files[0];
if (file) {
const reader = new FileReader();
reader.onload = this.handleReaderLoaded.bind(this);
reader.readAsBinaryString(file);
}
}
handleReaderLoaded(e) {
this.base64textString.push('data:image/png;base64,' + btoa(e.target.result));
}
HTML file
<input type="file" (change)="onUploadChange($event)" accept=".png, .jpg, .jpeg, .pdf" />
<img *ngFor="let item of base64textString" src={{item}} alt="" id="img">
Solution 3
another solution thats works for base64 is something like this post https://stackoverflow.com/a/36281449/6420568
in my case, i did
getImagem(readerEvt, midia){
//console.log('change no input file', readerEvt);
let file = readerEvt.target.files[0];
var reader = new FileReader();
reader.readAsDataURL(file);
reader.onload = function () {
//console.log('base64 do arquivo',reader.result);
midia.binario = btoa(reader.result);
//console.log('base64 do arquivo codificado',midia.binario);
};
reader.onerror = function (error) {
console.log('Erro ao ler a imagem : ', error);
};
}
and html component
<input type="file" class="form-control" (change)="getImagem($event, imagem)">
<img class="img-responsive" src="{{imagem.binario | decode64 }}" alt="imagem..." style="width: 200px;"/>
to display the image, i created the pipe decode64
@Pipe({
name: 'decode64'
})
export class Decode64Pipe implements PipeTransform {
transform(value: any, args?: any): any {
let a = '';
if(value){
a = atob(value);
}
return a;
}
}
Solution 4
Have you tried using btoa or Crypto.js to encode the image to base64 ?
link to cryptojs - https://code.google.com/archive/p/crypto-js/
var imgSrcData = window.btoa(fileLoadedEvent.target.result);
or
var imgSrcData = CryptoJS.enc.Base64.stringify(fileLoadedEvent.target.result);
Solution 5
Here is the same code from Parth Ghiya but written in ES6/TypeScript format
picture: string;
handleFileSelect(evt){
const file = evt.target.files[0];
if (!file) {
return false;
}
const reader = new FileReader();
reader.onload = () => {
this.picture = reader.result as string;
};
console.log(btoa(this.picture));
}
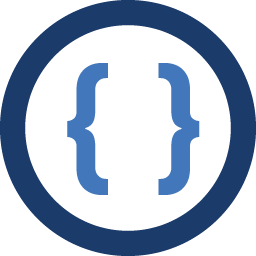
Admin
Updated on July 17, 2022Comments
-
Admin almost 2 years
Converting an image to base64 in angular 2, image is uploaded from local . Current am using fileLoadedEvent.target.result. The problem is, when I send this base64 string through REST services to java, it is not able to decode it. When i try this base64 string with free online encoder-decoder, there also I cannot see decoded image. I tried using canvas also. Am not getting proper result. One thing is sure the base64 string what am getting is not proper one, do I need to add any package for this ? Or in angular 2 is there any perticular way to encode the image to base64 as it was there in angular 1 - angular-base64-upload package.
Pls find below my sample code
onFileChangeEncodeImageFileAsURL(event:any,imgLogoUpload:any,imageForLogo:any,imageDiv:any) { var filesSelected = imgLogoUpload.files; var self = this; if (filesSelected.length > 0) { var fileToLoad = filesSelected[0]; //Reading Image file, encode and display var reader: FileReader = new FileReader(); reader.onloadend = function(fileLoadedEvent:any) { //SECOND METHO var imgSrcData = fileLoadedEvent.target.result; // <--- data: base64 var newImage = imageForLogo; newImage.src = imgSrcData; imageDiv.innerHTML = newImage.outerHTML; } reader.readAsDataURL(fileToLoad); } }