Disable return key in UITextView
14,872
Solution 1
Try to use like this..
- (BOOL)textView:(UITextView *)textView shouldChangeTextInRange:(NSRange)range replacementText:(NSString *)text {
if([text isEqualToString:@"\n"])
{
[textView resignFirstResponder];
return NO;
}
return YES;
}
Solution 2
Another solution without magic hardcoded strings will be:
- (BOOL)textView:(UITextView *)textView shouldChangeTextInRange:(NSRange)range replacementText: (NSString *)text {
if( [text rangeOfCharacterFromSet:[NSCharacterSet newlineCharacterSet]].location == NSNotFound ) {
return YES;
}
return NO;
}
Solution 3
In Swift 3+ add:
func textView(_ textView: UITextView, shouldChangeTextIn range: NSRange, replacementText text: String) -> Bool {
guard text.rangeOfCharacter(from: CharacterSet.newlines) == nil else {
// textView.resignFirstResponder() // uncomment this to close the keyboard when return key is pressed
return false
}
return true
}
Don't forget to add textView
's delegate in order for this to be called
Related videos on Youtube
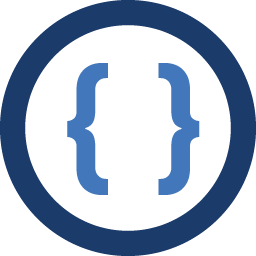
Author by
Admin
Updated on September 15, 2022Comments
-
Admin over 1 year
I am trying to disable the return key found when typing in a
UITextView
. I want the text to have no page indents like found in aUITextField
. This is the code I have so far:- (BOOL)textView:(UITextView *)aTextView shouldChangeTextInRange:(NSRange)aRange replacementText:(NSString*)aText { if ([aTextView.text isEqualToString:@"\r\n"]) { return NO; }
Any ideas?
-
Oliver Atkinson over 10 years+1, I like the idea of not using magic strings, which could change (they wont, but you catch my drift); It also reads easier.
-
Rupert almost 10 yearsThis doesn't take into account all possible line ending characters, or the fact that a user may paste a chunk of text in one go into the text field (so not equal to "\n" but containing it) - Grzegorz's solution is much more robust.
-
Rupert almost 10 yearsJust to clarify, I refer to the UITextview answer rather than the UITextField answers.