Does flask jsonify support UTF-8?
You are correct, jsonify does not support UTF-8 characters. It does, however, support Unicode characters perfectly well.
Consider these two programs:
# http server
from flask import Flask, jsonify
app = Flask(__name__)
@app.route('/')
def root():
return jsonify({'quote':'Birgün'})
if __name__=='__main__':
app.run(debug=True)
# http client
import requests
import unicodedata
r = requests.get('http://localhost:5000/')
j = r.json()
u = j['quote'][4]
print("%s: %d %x %s\n"%(u, len(u), ord(u), unicodedata.name(u)))
As you can see, the http client fetches the JSON, decodes it, and checks the "ü" in "Birgün".
The result should make it clear that the ü survived the end-to-end trip, from a Python3 string, through JSON and HTTP, and back into a Python3 string.
ü: 1 fc LATIN SMALL LETTER U WITH DIAERESIS
EDIT: Having said all of that, there is a configuration option which will force jsonify()
to behave as you hope:
app.config['JSON_AS_ASCII'] = False
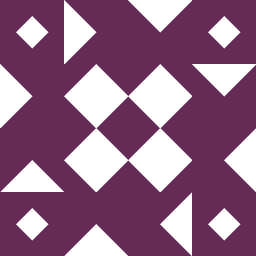
Comments
-
Ali 4 months
I've been doing experiments using flasks and jsonify. It works. But doesn't support utf-8 chararacters (turkish characters). I'm using a dictionary.
if api_key in key_list: quotes = { 'ataturk': ['Hayatta En Hakiki Mursit Ilimdir Fendir', 'Birgün benim naciz bedenim'], 'mahatma gandhi': ['Happiness is when what you think, what you' 'say,and what you do are in harmony.'] } get_quote = quotes[karakter(author.lower(), harfler)] quote = { 'quotes': random.choice(get_quote), } return jsonify(quote)
I've tried encode but it's not working. I got this error in debug mode:
AttributeError: 'dict' object has no attribute 'encode'
How can I solve this problem?
-
Ali over 6 yearsI guess I misunderstood. My output like: Birg\u00fcn benim naciz bedenim
-
alexisdevarennes over 6 yearsjsonify does not take a dict as a first argument, but it takes *args where each argument is the key to create in the json response. You're getting that error because jsonify is trying to run encode on a dict which is obviously not possible.
-
alexisdevarennes over 6 yearsI have updated the answer to have the complete example. Please try that, it should work.
-
Ali over 6 yearsWhat's your suggestions for dict alternative? My problem with character set. I thinking jsonify not supported utf-8 chars.
-
alexisdevarennes over 6 yearsSee my answer. Use jsonify(quotes=random.choice(get_quote))
-
Ali over 6 yearsmy codes works. My problem with character set. I thinking jsonify not supported utf-8 chars. Sample below: Birg\u00fcn benim naciz bedenim \u00fcn i dont want this. \u00fcn == ü
-
Ali over 6 yearsLet us continue this discussion in chat.
-
alexisdevarennes over 6 yearsIt really isn't that. You're passing a dict, just change the line with the return.
-
Ali over 6 yearsI've tried your code. I'm sorry. You did not understand me. I've tried avoid this: i.hizliresim.com/y5pkGa.png
-
alexisdevarennes over 6 yearsI've updated my answer. It was confusing, this is generally not the best thing to do though. You should iterate over the values and encode them. Otherwise I'd use ujson and set the content type header.
-
Ali over 6 yearsInteresting. Because I've tried this code:
print(sys.getdefaultencoding()) # output: utf-8
-
akashbw over 6 yearsthe change in config option worked for me :) Is there any downside for using this approach?