EOF when using pexpect and pxssh
Solution 1
Regarding #2: Expecting EOF is a red herring here. You -don't- expect EOF on login, you expect a password prompt on login. pxssh kicks that error when it gets back EOF on login from ssh without getting a password prompt. This can happen because it's using ssh -q to not get warnings, and you're getting a warning from ssh. Take the ssh options it is using and run them yourself without the q:
/usr/bin/ssh -l root 127.0.0.1
In my case, I can get this error message when ssh is kicking out a known hosts violation due to the machine I am connecting to having its identity changed.
Solution 2
I had the same error while trying to use self.expect()
in pxssh
. Changing the ssh options by removing the '-q' did not work for me.
I followed the instructions in this site by adding pexpect.EOF as one of the inputs to self.expect()
. This will make the script wait till the entire string is received before exiting when EOF is received:
i = self.expect(["(?i)are you sure you want to continue connecting", original_prompt, "(?i)(?:password.*)|(?:passphrase for key)", "(?i)permission denied", "(?i)terminal type", pexpect.EOF, TIMEOUT, "(?i)connection closed by remote host"], timeout=login_timeout)
With this, it works fine !
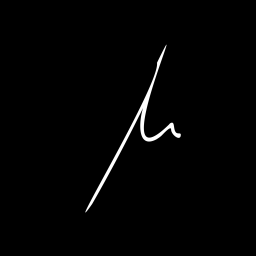
mbaytas
Updated on October 31, 2022Comments
-
mbaytas less than a minute
I'm trying to run the code in the Interacting with SSH Through Pexpect and Brute Forcing SSH Passwords with Pxssh sections from Chapter 2 of Violent Python. Using both
child.expect()
andpxssh
I get similar EOF errors.Running these commands from the Python console:
import pexpect connStr = "ssh [email protected]" child = pexpect.spawn(connStr) ret = child.expect([pexpect.TIMEOUT, ssh_newkey, "[P|p]assword:"])
I get this output:
Traceback (most recent call last): File "<input>", line 1, in <module> File "/Library/Frameworks/Python.framework/Versions/2.7/lib/python2.7/site-packages/pexpect.py", li ne 1316, in expect return self.expect_list(compiled_pattern_list, timeout, searchwindowsize) File "/Library/Frameworks/Python.framework/Versions/2.7/lib/python2.7/site-packages/pexpect.py", li ne 1330, in expect_list return self.expect_loop(searcher_re(pattern_list), timeout, searchwindowsize) File "/Library/Frameworks/Python.framework/Versions/2.7/lib/python2.7/site-packages/pexpect.py", li ne 1401, in expect_loop raise EOF (str(e) + '\n' + str(self)) EOF: End Of File (EOF) in read_nonblocking(). Empty string style platform. <pexpect.spawn object at 0x10180c550> version: 2.4 ($Revision: 516 $) command: /usr/bin/ssh args: ['/usr/bin/ssh', '[email protected]'] searcher: searcher_re: 0: TIMEOUT 1: re.compile("Are you sure you want to continue connecting") 2: re.compile("[P|p]assword:") buffer (last 100 chars): before (last 100 chars): after: <class 'pexpect.EOF'> match: None match_index: None exitstatus: 255 flag_eof: True pid: 12122 child_fd: 4 closed: False timeout: 30 delimiter: <class 'pexpect.EOF'> logfile: None logfile_read: None logfile_send: None maxread: 2000 ignorecase: False searchwindowsize: None delaybeforesend: 0.05 delayafterclose: 0.1 delayafterterminate: 0.1
And running these commands, using
pxssh
:import pxssh s = pxssh.pxssh() s.login("127.0.0.1", "root", "1234")
I get this output:
Traceback (most recent call last): File "<input>", line 1, in <module> File "/Library/Frameworks/Python.framework/Versions/2.7/lib/python2.7/site-packages/pxssh.py", line 196, in login i = self.expect(["(?i)are you sure you want to continue connecting", original_prompt, "(?i)(?:pas sword)|(?:passphrase for key)", "(?i)permission denied", "(?i)terminal type", TIMEOUT, "(?i)connectio n closed by remote host"], timeout=login_timeout) File "/Library/Frameworks/Python.framework/Versions/2.7/lib/python2.7/site-packages/pexpect.py", li ne 1316, in expect return self.expect_list(compiled_pattern_list, timeout, searchwindowsize) File "/Library/Frameworks/Python.framework/Versions/2.7/lib/python2.7/site-packages/pexpect.py", li ne 1330, in expect_list return self.expect_loop(searcher_re(pattern_list), timeout, searchwindowsize) File "/Library/Frameworks/Python.framework/Versions/2.7/lib/python2.7/site-packages/pexpect.py", li ne 1401, in expect_loop raise EOF (str(e) + '\n' + str(self)) EOF: End Of File (EOF) in read_nonblocking(). Empty string style platform. <pxssh.pxssh object at 0x1016bff90> version: 2.4 ($Revision: 516 $) command: /usr/bin/ssh args: ['/usr/bin/ssh', '-q', '-l', 'root', '127.0.0.1'] searcher: searcher_re: 0: re.compile("(?i)are you sure you want to continue connecting") 1: re.compile("[#$]") 2: re.compile("(?i)(?:password)|(?:passphrase for key)") 3: re.compile("(?i)permission denied") 4: re.compile("(?i)terminal type") 5: TIMEOUT 6: re.compile("(?i)connection closed by remote host") buffer (last 100 chars): before (last 100 chars): after: <class 'pexpect.EOF'> match: None match_index: None exitstatus: None flag_eof: True pid: 12136 child_fd: 3 closed: False timeout: 30 delimiter: <class 'pexpect.EOF'> logfile: None logfile_read: None logfile_send: None maxread: 2000 ignorecase: False searchwindowsize: None delaybeforesend: 0.05 delayafterclose: 0.1 delayafterterminate: 0.1
I get similar results when I substitute 127.0.0.1 with other hosts and try different username/password combinations.
The pexpect documentation suggests using
expect(pexpect.EOF)
to avoid generating the EOF exception. Indeed, when I do the following:connStr = "ssh [email protected]" child = pexpect.spawn(connStr) print child.expect(pexpect.EOF)
The result is
0
.But the following questions remain:
- I'm confused by the book's syntax:
child.expect([pexpect.TIMEOUT, ssh_newkey, "[P|p]assword:"])
. Why are we passing a list toexpect()
? What is this list supposed to contain? - How do I make use of
expect(pexpect.EOF)
, as the documentation instructs, when using pxssh? - Why does the code in the book not work properly? Has something changed in the pexpect library since the book's publication? Is it because I am on OS X?
I have Python 2.7 and pexpect 2.4 running on Mac OS X 10.8.4.
- I'm confused by the book's syntax: