Extract string within parentheses - PYTHON
31,956
Solution 1
You can use a simple regex to catch everything between the parenthesis:
>>> import re
>>> s = 'Name(something)'
>>> re.search('\(([^)]+)', s).group(1)
'something'
The regex matches the first "(", then it matches everything that's not a ")":
\(
matches the character "(" literally- the capturing group
([^)]+)
greedily matches anything that's not a ")"
Solution 2
as an improvement on @Maroun Maroun 's answer:
re.findall('\(([^)]+)', s)
it finds all instances of strings in between parentheses
Solution 3
You can use split as in your example but this way
val = s.split('(', 1)[1].split(')')[0]
or using regex
Solution 4
You can use re.match
:
>>> import re
>>> s = "name(something)"
>>> na, so = re.match(r"(.*)\((.*)\)" ,s).groups()
>>> na, so
('name', 'something')
that matches two (.*)
which means anything, where the second is between parentheses \(
& \)
.
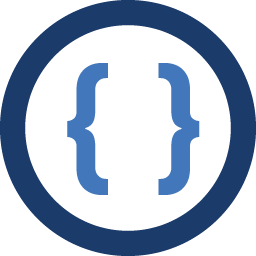
Author by
Admin
Updated on July 09, 2022Comments
-
Admin almost 2 years
I have a string "Name(something)" and I am trying to extract the portion of the string within the parentheses!
Iv'e tried the following solutions but don't seem to be getting the results I'm looking for.
n.split('()') name, something = n.split('()')
-
Admin over 7 yearsThanks! makes sense, however i'm getting the error that re is not defined, do you need to import a library for this function?
-
Maroun over 7 years@olly_t
import re
-
Bharath Kumar over 2 yearsi/p cmd = (0, 45, -4, -9) after print('cmd =',cmd.split('(', 1)[1].split(')')[0]) o/p cmd = 0, 45, -4, -9