How to extract the first and final words from a string?
Solution 1
You have to firstly convert the string to list
of words using str.split
and then you may access it like:
>>> my_str = "Hello SO user, How are you"
>>> word_list = my_str.split() # list of words
# first word v v last word
>>> word_list[0], word_list[-1]
('Hello', 'you')
From Python 3.x, you may simply do:
>>> first, *middle, last = my_str.split()
Solution 2
If you are using Python 3, you can do this:
text = input()
first, *middle, last = text.split()
print(first, last)
All the words except the first and last will go into the variable middle
.
Solution 3
Let's say x
is your input. Then you may do:
x.partition(' ')[0]
x.partition(' ')[-1]
Solution 4
You would do:
print text.split()[0], text.split()[-1]
Solution 5
Some might say, there is never too many answer's using regular expressions (in this case, this looks like the worst solutions..):
>>> import re
>>> string = "Hello SO user, How are you"
>>> matches = re.findall(r'^\w+|\w+$', string)
>>> print(matches)
['Hello', 'you']
Related videos on Youtube
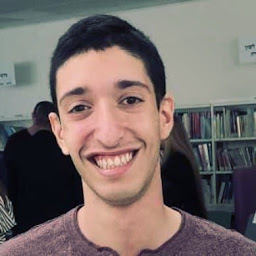
Lior Dahan
10th grade student from Israel. I will destroy each and every one of you in CSGO
Updated on November 21, 2021Comments
-
Lior Dahan over 2 years
I have a small problem with something I need to do in school...
My task is the get a raw input string from a user (
text = raw_input()
) and I need to print the first and final words of that string.Can someone help me with that? I have been looking for an answer all day...
-
Moinuddin Quadri over 7 yearsBy final word do you mean last word? Please mention the sample example
-
John Coleman over 7 yearsIs the last word in
"Hello World!"
the string"World!"
or the word"World"
?
-
-
wildwilhelm over 7 years
partition
will only chopx
once (so, you'll get the first word, and then the rest of the sentence, not the last word). -
Moinuddin Quadri over 7 years@wildwilhelm: There is a possibility that this answer is what OP expects as there is no clarity on the definition of final words. As a matter of doubt, answer should not be down-voted
-
wildwilhelm over 7 yearsTrue, I agree that the question wording is ambiguous. Downvote retracted.
-
quapka over 7 yearsCool, first time I see this feature.
-
Moinuddin Quadri over 7 yearsNice. If middle words were also required, it is very clean approach
-
John Coleman over 7 yearsThis is nice, though it fails in the edge case of a 1-word sentence (where arguably that 1 word should be returned as both the first and the last word).
-
PatrickT almost 4 years@JohnColeman, In this case, it errors out with
ValueError: not enough values to unpack
, which IMHO is a reasonable interpretation of "cannot split a word at a whitespace when such word does not have whitespace". -
Tomerikoo about 2 yearsWhat's not clear about first and final? This answer will only give the first and second words, no the final if there are more than two words...