Filling array in C by using functions
Solution 1
Assuming that input_data
should set all array values to a known value, you could write
void input_data(int *data, int size, int value){
for (int i=0; i<size; i++) {
data[i] = value;
}
}
calling this like
input_data(*data1, *size1, 5); // set all elements of data1 to 5
The key point here is that you can use (*data1)[index]
to access a particular array element and can pass your arrays as int*
arguments.
Solution 2
I stumbled upon this question while doing a homework assignment and the answer doesn't strike me as entirely satisfactory, so I'll try to improve upon it.
Here is a small program that will establish an array of a user-defined size, fill it arbitrarily, and print it.
#include <stdio.h>
#include <stdlib.h>
void fill_array(int *array, int n)
{
int i;
for (i = 0; i < n; i++)
{
// fill array like [1, 2, 3, 4...]
array[i] = i+1;
}
}
void print_array(int *array, int n)
{
int i;
printf("[");
for (i = 0; i < n; i++)
{
if (i == (n-1))
printf("%d]\n", array[i]);
else
printf("%d, ", array[i]);
}
}
int main()
{
int n;
printf("Please enter a size for your array>");
scanf("%d", &n);
// dynamically allocate memory for integer array of size n
array = (int*) malloc (n * (sizeof(int)));
fill_array(array, n);
print_array(array, n);
return 0;
}
I hope this helps anyone who is learning C for the first time, or coming back to it after years away from the tried and true language, like me.
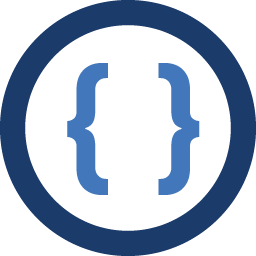
Admin
Updated on July 05, 2022Comments
-
Admin almost 2 years
Okay, so I am calling function fill_arrays like this:
fill_arrays(&data1, &data2, &size1, &size2);
fill_arrays looks like this:
void fill_arrays(int **data1, int **data2, int *size1, int *size2){ *size1 = get_size(*size1, 1); *size2 = get_size(*size2, 2); *data1 = malloc(*size1 * sizeof(int *)); *data2 = malloc(*size2 * sizeof(int *)); input_data(&data1, *size1, 1); }
In input_data function I would like to assign some numbers to an array:
void input_data(int **data, int size, int index){ *data[5] = 5; }
The problem is, I am completely lost with pointers... Maybe you can tell me how should I call function input_data in order to be able to assign some numbers to data array?