Finding intersection of two lists of strings in python
Solution 1
You can use flatten
function of compiler.ast
module to flatten your sub-list and then apply set intersection like this
from compiler.ast import flatten
A=[['11@N3'], ['23@N0'], ['62@N0'], ['99@N0'], ['47@N7']]
B=[['23@N0'], ['12@N1']]
a = flatten(A)
b = flatten(B)
common_elements = list(set(a).intersection(set(b)))
common_elements
['23@N0']
Solution 2
The problem is that your lists contain sublists so they cannot be converted to sets. Try this:
A=[['11@N3'], ['23@N0'], ['62@N0'], ['99@N0'], ['47@N7']]
B=[['23@N0'], ['12@N1']]
C = [item for sublist in A for item in sublist]
D = [item for sublist in B for item in sublist]
print set(C).intersection(set(D))
Solution 3
Your datastructure is a bit strange, as it is a list of one-element lists of strings; you'd want to reduce it to a list of strings, then you can apply the previous solutions:
Thus a list like:
B = [['23@N0'], ['12@N1']]
can be converted to iterator that iterates over '23@N0', '12@N1'
with itertools.chain(*)
, thus we have simple oneliner:
>>> set(chain(*A)).intersection(chain(*B))
{'23@N0'}
Solution 4
In case you have to fit it on a fortune cookie:
set(i[0] for i in A).intersection(set(i[0] for i in B))
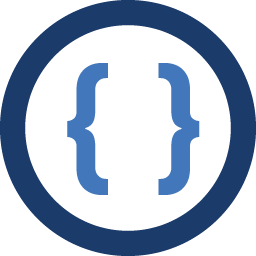
Admin
Updated on July 30, 2022Comments
-
Admin over 1 year
I have gone through Find intersection of two lists?, Intersection of Two Lists Of Strings, Getting intersection of two lists in python. However, I could not solve this problem of finding intersection between two string lists using Python.
I have two variables.
A = [['11@N3'], ['23@N0'], ['62@N0'], ['99@N0'], ['47@N7']] B = [['23@N0'], ['12@N1']]
How to find that '23@N0' is a part of both A and B?
I tried using intersect(a,b) as mentioned in http://www.saltycrane.com/blog/2008/01/how-to-find-intersection-and-union-of/ But, when I try to convert A into set, it throws an error:
File "<stdin>", line 1, in <module> TypeError: unhashable type: 'list'
To convert this into a set, I used the method in TypeError: unhashable type: 'list' when using built-in set function where the list can be converted using
result = sorted(set(map(tuple, A)), reverse=True)
into a tuple and then the tuple can be converted into a set. However, this returns a null set as the intersection.
Can you help me find the intersection?