Firebase Messaging notification for specific device in flutter
Solution 1
I have read some implementations for what you are trying to achieve and I guess you should follow this steps:
- Install Firebase Cloud Messaging (FCM) for Flutter (I see you already done it)
- Get the device firebase messaging token by calling the "getToken" method provided by FCM
- Save this token when user logs in on a document of Firebase Cloud Firestore to associate this device token to the user (or create one array of tokens for the user so you can have multiple devices per user
- Monitor when messaging happens on your database. When it happens, gather the destination user, get the token(s) associated to this user and send one FCM to that specific device id(s). For this point, I suggest you use Firebase Cloud Functions despite all the duplication of data between servers that this may entail (ex: your server has the user name with some id and that same id "lives" in some noSQL document inside Firestore)
Hope it helps. I provide no code for any of this because I believe it is not what you are searching for.
Solution 2
You need change
'to': await firebaseMessaging.getToken(),
to
'to': '${token of specific devices}',
.
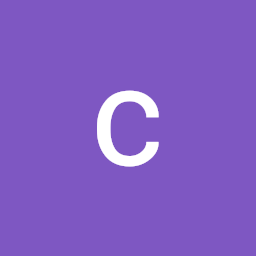
custom apps
Updated on December 15, 2022Comments
-
custom apps over 1 year
I am working on a flutter application whereby a lot of server requests were implemented on our custom server including login/signup. However, we decided to use Firebase Cloud Messaging for notifications. For example, included in the application is a chat feature.
During end to end messaging, as messages get posted to our server, users should receive notifications on their specific devices. I have already successfully implemented and configured
firebase
within the app and can send messages from the console but i need to make it work on specific devices.// Replace with server token from firebase console settings. final String serverToken = '<Server-Token>'; final FirebaseMessaging firebaseMessaging = FirebaseMessaging(); Future<Map<String, dynamic>> sendAndRetrieveMessage() async { await firebaseMessaging.requestNotificationPermissions( const IosNotificationSettings(sound: true, badge: true, alert: true, provisional: false), ); await http.post( 'https://fcm.googleapis.com/fcm/send', headers: <String, String>{ 'Content-Type': 'application/json', 'Authorization': 'key=$serverToken', }, body: jsonEncode( <String, dynamic>{ 'notification': <String, dynamic>{ 'body': 'this is a body', 'title': 'this is a title' }, 'priority': 'high', 'data': <String, dynamic>{ 'click_action': 'FLUTTER_NOTIFICATION_CLICK', 'id': '1', 'status': 'done' }, 'to': await firebaseMessaging.getToken(), }, ), );
This is the documented way of sending messages within flutter. However, this will send notifications to all users not a single user. So, how do i target a specific user?