Flutter DataTable select Row
7,275
Solution 1
You can copy past run full code below
You can use condition in selected
like this 0 == selectedIndex
where 0 is DataRow
index
and change selectedIndex
in onSelectChanged
code snippet
int selectedIndex = -1;
...
rows: [
DataRow(
selected: 0 == selectedIndex,
onSelectChanged: (val) {
setState(() {
selectedIndex = 0;
});
},
...
DataRow(
selected: 1 == selectedIndex,
onSelectChanged: (val) {
setState(() {
selectedIndex = 1;
});
},
working demo
full code
import 'package:flutter/material.dart';
void main() {
runApp(MyApp());
}
class MyApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Flutter Demo',
theme: ThemeData(
primarySwatch: Colors.blue,
visualDensity: VisualDensity.adaptivePlatformDensity,
),
home: MyHomePage(title: 'Flutter Demo Home Page'),
);
}
}
class MyHomePage extends StatefulWidget {
MyHomePage({Key key, this.title}) : super(key: key);
final String title;
@override
_MyHomePageState createState() => _MyHomePageState();
}
class _MyHomePageState extends State<MyHomePage> {
Color color;
int selectedIndex = -1;
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: Text(widget.title),
),
body: Center(
child: Column(
children: <Widget>[
DataTable(
onSelectAll: (val) {
setState(() {
selectedIndex = -1;
});
},
columns: [
DataColumn(label: Text('Language')),
DataColumn(label: Text('Translation')),
],
rows: [
DataRow(
selected: 0 == selectedIndex,
onSelectChanged: (val) {
setState(() {
selectedIndex = 0;
});
},
cells: [
DataCell(
Text(
"RO",
textAlign: TextAlign.left,
style: TextStyle(fontWeight: FontWeight.bold),
),
onTap: () {
setState(() {
color = Colors.lightBlueAccent;
});
},
),
DataCell(
TextField(
decoration: InputDecoration(
border: InputBorder.none, hintText: 'paine'),
),
),
]),
DataRow(
selected: 1 == selectedIndex,
onSelectChanged: (val) {
setState(() {
selectedIndex = 1;
});
},
cells: [
DataCell(
Text(
"EN",
textAlign: TextAlign.left,
style: TextStyle(fontWeight: FontWeight.bold),
), onTap: () {
print('EN is clicked');
}),
DataCell(
TextField(
decoration: InputDecoration(
border: InputBorder.none, hintText: 'bread'),
),
),
]),
DataRow(
selected: 2 == selectedIndex,
onSelectChanged: (val) {
setState(() {
selectedIndex = 2;
});
},
cells: [
DataCell(
Text(
"FR",
textAlign: TextAlign.left,
style: TextStyle(fontWeight: FontWeight.bold),
), onTap: () {
print('FR is clicked');
}),
DataCell(
TextField(
decoration: InputDecoration(
border: InputBorder.none, hintText: 'pain'),
),
),
]),
],
),
],
),
),
);
}
}
Solution 2
When I needed to call something when I pressed on the DataRow
I did the following:
DataRow(
onSelectChanged(_) {
// do something here
}
cells: [],
),
Maybe this will help?
EDIT: this is a simple example of how to select/deselect rows
import 'package:flutter/material.dart';
class Stack1 extends StatefulWidget {
@override
_Stack1State createState() => _Stack1State();
}
class _Stack1State extends State<Stack1> {
bool row1Selected = false;
bool row2Selected = false;
@override
Widget build(BuildContext context) {
return Scaffold(
body: DataTable(
columns: [
DataColumn(
label: Text('My Data'),
),
],
rows: [
DataRow(
selected: row1Selected,
onSelectChanged: (value) {
setState(() {
row1Selected = value;
});
},
cells: [
DataCell(
Text('Data 1'),
),
],
),
DataRow(
selected: row2Selected,
onSelectChanged: (value) {
setState(() {
row2Selected = value;
});
},
cells: [
DataCell(
Text('Data 2'),
),
],
),
],
),
);
}
}
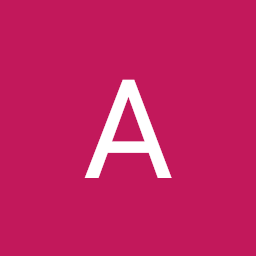
Author by
Alexandru Buruiana
Updated on December 23, 2022Comments
-
Alexandru Buruiana over 1 year
I have a problem with DataTable in flutter. I want to change the state of the row, when the user click on one of them, but I found just how to do this "manually" with selected: true, inside DataRow.
How can I let the user select just a single row, and deselect the rest, by changing the state?
content: Column( children: <Widget>[ DataTable( columns: [ DataColumn(label: Text('Language')), DataColumn(label: Text('Translation')), ], rows: [ DataRow(selected: true, cells: [ DataCell( Text( "RO", textAlign: TextAlign.left, style: TextStyle(fontWeight: FontWeight.bold), ), onTap: () { setState(() { color = Colors.lightBlueAccent; }); }, ), DataCell( TextField( decoration: InputDecoration( border: InputBorder.none, hintText: 'paine'), ), ), ]), DataRow(cells: [ DataCell( Text( "EN", textAlign: TextAlign.left, style: TextStyle(fontWeight: FontWeight.bold), ), onTap: () { print('EN is clicked'); }), DataCell( TextField( decoration: InputDecoration( border: InputBorder.none, hintText: 'bread'), ), ), ]), DataRow(cells: [ DataCell( Text( "FR", textAlign: TextAlign.left, style: TextStyle(fontWeight: FontWeight.bold), ), onTap: () { print('FR is clicked'); }), DataCell( TextField( decoration: InputDecoration( border: InputBorder.none, hintText: 'pain'), ), ), ]), ], ), ], ),
-
Alexandru Buruiana over 3 yearsAnd how could I change the "selected" mode inside the method? Or to change the color of the DataRow, anything would be useful..
-
Matthew Trent over 3 years@AlexandruBuruiana I'm not sure at the moment, I'll try to find a solution right now.