Flutter error: No TabController for TabBarView
Solution 1
use SingleTickerProviderStateMixin
. You have just one controller.
And there is no any problem I passed your code dartpad it worked properly. Check your other codes.
Just open https://dartpad.dartlang.org/flutter and paste these codes.
import 'package:flutter/material.dart';
final Color darkBlue = Color.fromARGB(255, 18, 32, 47);
void main() {
runApp(MyApp());
}
class MyApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
theme: ThemeData.dark().copyWith(scaffoldBackgroundColor: darkBlue),
debugShowCheckedModeBanner: false,
home: Scaffold(
body: Center(
child: ProductBody(),
),
),
);
}
}
class ProductBody extends StatefulWidget {
@override
_ProductBodyState createState() => _ProductBodyState();
}
class _ProductBodyState extends State<ProductBody> with TickerProviderStateMixin{
TabController _controller;
@override
void initState() {
_controller = new TabController(length: 2, vsync: this);
super.initState();
}
@override
Widget build(BuildContext context) {
return Container(
padding: EdgeInsets.only(top: 20.0, left: 20.0, right: 20.0),
child: SingleChildScrollView(
scrollDirection: Axis.vertical,
child: Column(
children: <Widget>[
Align(
alignment: Alignment.centerLeft,
child: Text(
"Select category:",
style: TextStyle(
color: Colors.green,
fontWeight: FontWeight.bold,
fontSize: 16,
),
),
),
Container(
decoration: new BoxDecoration(color: Theme.of(context).primaryColor),
child: new TabBar(
controller: _controller,
tabs: [
Tab(
icon: const Icon(Icons.home),
text: 'Address',
),
Tab(
icon: const Icon(Icons.my_location),
text: 'Location',
),
],
),
),
Container(
height: 80.0,
child: new TabBarView(
controller: _controller,
children: <Widget>[
new Card(
child: new ListTile(
leading: const Icon(Icons.home),
title: new TextField(
decoration: const InputDecoration(hintText: 'Search for address...'),
),
),
),
new Card(
child: new ListTile(
leading: const Icon(Icons.location_on),
title: new Text('Latitude: 48.09342\nLongitude: 11.23403'),
trailing: new IconButton(icon: const Icon(Icons.my_location), onPressed: () {}),
),
),
],
),
),
],
),
),
);
}
}
Solution 2
You can use bottom Navigation tabs. The tab bar will appear in the bottom of the page, and works just like a default tab bar
https://medium.com/@uncoded_decimal/creating-bottom-navigation-tabs-using-flutter-2286681450d4
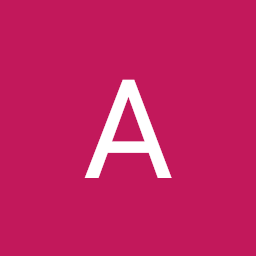
Alexandru Buruiana
Updated on December 24, 2022Comments
-
Alexandru Buruiana over 1 year
I want to add some tabs in my flutter page, but without having a page just with tabs.
For short, I have some input fields, and underneath them I want to put my Tabs.
I keep getting this error: No TabController for TabBarView. I already gave the state class to be with TickerProviderStateMixin for the controller to work, but I can't figure out why it says that no tabcontroller is found, when it exists.
The problem is that I already have a tab controller, this is the code:
class ProductBody extends StatefulWidget { @override _ProductBodyState createState() => _ProductBodyState(); } class _ProductBodyState extends State<ProductBody> with TickerProviderStateMixin{ TabController _controller; @override void initState() { _controller = new TabController(length: 2, vsync: this); super.initState(); } @override Widget build(BuildContext context) { return Container( padding: EdgeInsets.only(top: 20.0, left: 20.0, right: 20.0), child: SingleChildScrollView( scrollDirection: Axis.vertical, child: Column( children: <Widget>[ Align( alignment: Alignment.centerLeft, child: Text( "Select category:", style: TextStyle( color: Colors.green, fontWeight: FontWeight.bold, fontSize: 16, ), ), ), Container( decoration: new BoxDecoration(color: Theme.of(context).primaryColor), child: new TabBar( controller: _controller, tabs: [ Tab( icon: const Icon(Icons.home), text: 'Address', ), Tab( icon: const Icon(Icons.my_location), text: 'Location', ), ], ), ), Container( height: 80.0, child: new TabBarView( controller: _controller, children: <Widget>[ new Card( child: new ListTile( leading: const Icon(Icons.home), title: new TextField( decoration: const InputDecoration(hintText: 'Search for address...'), ), ), ), new Card( child: new ListTile( leading: const Icon(Icons.location_on), title: new Text('Latitude: 48.09342\nLongitude: 11.23403'), trailing: new IconButton(icon: const Icon(Icons.my_location), onPressed: () {}), ), ), ], ), ), ], ), ), ); } }
-
Alexandru Buruiana over 3 yearsI changed the code with
SingleTickerProviderStateMixin
, same error:No TabController for TabBar.
-
Mr. ZeroOne over 3 yearsThe problem is not there. I passed your code in dartpad it worked properly.
-
Alexandru Buruiana over 3 yearsCan you give me the link for the dartpad?
-
Mr. ZeroOne over 3 yearsUnfortunatly We can't share codes with dartpad. Just open dartpad.dartlang.org/flutter and past your code. Replace the ProductBody widget with MyWidget.
-
Alexandru Buruiana over 3 yearsIt's not working, it has a lot of errors:
Type 'StatefulWidget' not found.
Type 'BuildContext' not found.
-
Alexandru Buruiana over 3 yearsI don't understand why, but I restarted my app, and now it work with no problem. It works with both
SingleTickerProviderStateMixin
andTickerProviderStateMixin
.