Flutter Show Modal Bottom Sheet after build
Solution 1
One of the solutions might be using addPostFrameCallback function of the SchedulerBinding instance. This way you could call showModalBottomSheet after the Home widget is built.
import 'package:flutter/scheduler.dart';
...
@override
Widget build(BuildContext context) {
SchedulerBinding.instance.addPostFrameCallback((timeStamp) {
showModalBottomSheet<void>(
context: context,
builder: (BuildContext context) {
//Your builder code
},
);
});
//Return widgets tree for Home
}
Solution 2
Here's one way:
class MyApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
WidgetsBinding.instance.addPostFrameCallback((timeStamp) {
showModalBottomSheet(
context: context,
builder: (BuildContext context) {
return Container(
child: Text('heyooo'),
);
}
);
});
return Scaffold(
appBar: AppBar(),
body: Container(),
);
}
}
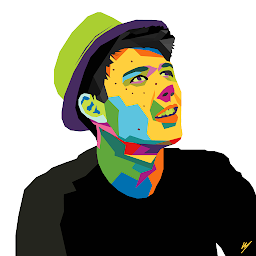
Leonardo Mantovani
I’m a student who likes spending his free time coding and learning new skills. I’ve attended a Coursera Specialization by the University of Colorado System for videogames development using Unity and C#. I’ve attended a course (and many tutorials) to learn Python too, especially to use Django Even though I’m still a beginner, I’ve already did some stuff, check it out on my website https://lezsoft.com
Updated on December 01, 2022Comments
-
Leonardo Mantovani over 1 year
As the title says, I have a String parameter and when I load the Home Stateful Widget I would like to open this bottom sheet if the parameter is not null.
As I understood I can't call
showModalBottomSheet()
in the build function of the Home widget because it can't start building the bottom sheet while building the Home Widget, so, is there a way to call this immediately after the Home Widget is built? -
Leonardo Mantovani over 3 yearsThank you, that's exactly what I was looking for