Flutter web - Uploading image to firebase storage
1,105
You can use the putData() method to send the image and set it's metadata as a image.
Future<String> uploadImage(PlatformFile file) async {
try {
TaskSnapshot upload = await FirebaseStorage.instance
.ref(
'events/${file.path}-${DateTime.now().toIso8601String()}.${file.extension}')
.putData(
file.bytes,
SettableMetadata(contentType: 'image/${file.extension}'),
);
String url = await upload.ref.getDownloadURL();
return url;
} catch (e) {
print('error in uploading image for : ${e.toString()}');
return '';
}
}
putData() method takes Uint8List by default.
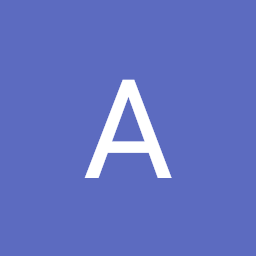
Author by
Arbaz Irshad
Updated on December 29, 2022Comments
-
Arbaz Irshad over 1 year
I am using the
firebase_storage: ^8.0.6
package on flutter web. I want to upload image to firebase storage that I get usingFilePicker
package. The problem is that the new package uses the putFile() method to upload files. But File from dart:io doesn't work on flutter web and it also doesn't accept the File object from dart:html.I can upload image as Blob using the putBlob() method but then it doesn't upload it as image type but it's type is
application/octet-stream
. I don't want to upload the image file as a blob.Future<String> uploadImage(PlatformFile file) async { try { TaskSnapshot upload = await FirebaseStorage.instance .ref( 'events/${file.name}-${DateTime.now().toIso8601String()}.${file.extension}') .putBlob(Blob(file.bytes)); String url = await upload.ref.getDownloadURL(); return url; } catch (e) { print('error in uploading image for : ${e.toString()}'); return '; } }
How to fix this issue?