Form submission after Javascript Validation
24,264
Solution 1
Just add action="reg.php"
in <form>
and return val()
functions on onclick
, also make type="submit"
to form submission, like:
HTML CODE:
<form method="post" action="reg.php">
<input type="text" id="username" name="username"></input>
<button type="submit" onclick="return val()">Sign Up</button>
</form>
JAVASCRIPT CODE:
Just add return true;
in else
like:
function val(){
var uname = document.getElementById('username').value;
if(!uname){
document.getElementById("error").innerHTML = "Enter a username";
return false; // in failure case
}
return true; // in success case
}
Solution 2
You can submit it with form name
in else section.
document.myForm.submit();
var uname = document.getElementById('username').value;
if (!uname) {
document.getElementById("error").innerHTML = "Enter a username";
return false;
} else {
document.myForm.submit(); // Form will be submitted by it's name
}
Markup
<form method="post" name="myForm">.....</form>
Demo
Solution 3
HTML Code
<form method="post" onsubmit="return val()">
<input type="text" id="username" name="username">
<button type="submit" >Sign Up</button>
</form>
Javascript Code
function val(){
var uname = document.getElementById('username').value;
if(!uname){
document.getElementById("error").innerHTML = "Enter a username";
return false;
}
else{
return true;
}
}
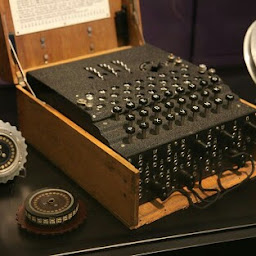
Author by
Enigma Crack
Updated on July 09, 2022Comments
-
Enigma Crack almost 2 years
I want to submit my form to a
PHP
file calledreg.php
after validating it usingJavaScript
. TheHTML
form is given below:HTML Form:
<form method="post"> <input type="text" id="username" name="username"></input> <button type="button" onclick="val()">Sign Up</button> </form>
JavaScript Validation
The following is the
JavaScript
validation code:function val(){ var uname = document.getElementById('username').value; if(!uname){ document.getElementById("error").innerHTML = "Enter a username"; return false; } else{ // I want to submit form if this else statement executes. } }