Function or fat arrow for a React functional component?
Solution 1
There is no practical difference.
An arrow allows to skip return
keyword, but cannot benefit from hoisting. This results in less verbose output with ES6 target but more verbose output when transpiled to ES5 , because a function is assigned to a variable:
var MyMainComponent = function MyMainComponent() {
return React.createElement(
"main",
null,
React.createElement("h1", null, "Welcome to my app")
);
};
The overhead is 6 bytes in minified and not gzipped JS file, this consideration can be generally ignored.
Verbosity is not necessarily the case when an arrow is exported, due to optimizations:
var MyMainComponent = (exports.MyMainComponent = function MyMainComponent() {
return React.createElement(
"main",
null,
React.createElement("h1", null, "Welcome to my app")
);
});
Solution 2
Mostly a matter of preference. However, there are some (minor, almost insignificant) differences:
The fat arrow syntax lets you omit the curly braces and the
return
keyword if you return the JSX directly, without any prior expressions. With ES5 functions, you must have the{ return ... }
.The fat arrow syntax does not create a new context of
this
, whereas ES5 functions do. This can be useful when you wantthis
inside the function to reference the React component or when you want to skip thethis.foo = this.foo.bind(this);
step.
There are more differences between them, but they are rarely relative when coding in React (e.g using arguments
, new
, etc).
On a personal note, I use the fat arrow syntax whenever possible, as I prefer that syntax.
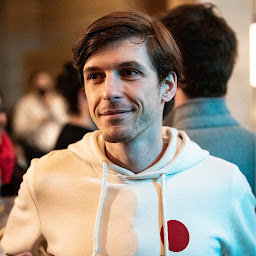
Dispix
Updated on July 17, 2022Comments
-
Dispix almost 2 years
I can't help but wondering if there's any advantage between using plain functions and fat arrows for React functional components
const MyMainComponent = () => ( <main> <h1>Welcome to my app</h1> </main> ) function MyMainComponent() { return ( <main> <h1>Welcome to my app</h1> </main> ) }
Both work of course perfectly fine but is there a recommended way to write those ? Any argument in favor of one or the other ?
Edit: I am aware of the differences when it comes to plain javascript functions (i.e. context, stack trace, return keyword, etc.) that can have an impact for the kind of use you have for functions. However I am asking the question purely in terms of React components.
-
Estus Flask over 5 yearsThe second item is not the case because functional components have no
this
. -
Chris over 5 years@estus, true. I was referring to the fat arrow syntax in general (e.g for methods).