getting emails out of string - regex syntax + preg_match_all
16,586
Solution 1
Like this
$pattern = '/[A-Z0-9._%+-][email protected][A-Z0-9.-]+\.[A-Z]{2,4}\b/i';
Or smaller version :)
$pattern = '/[a-z\d._%+-][email protected][a-z\d.-]+\.[a-z]{2,4}\b/i';
Solution 2
When using PCRE regex functions it require to enclose the pattern by delimiters:
Often used delimiters are forward slashes (/), hash signs (#) and tildes (~). The following are all examples of valid delimited patterns.
/foo bar/
#^[^0-9]$#
+php+
%[a-zA-Z0-9_-]%
Then you must correct this line to:
$pattern = '/\b[A-Z0-9._%+-][email protected][A-Z0-9.-]+\.[A-Z]{2,4}\b/';
or
$pattern = '#\b[A-Z0-9._%+-][email protected][A-Z0-9.-]+\.[A-Z]{2,4}\b#';
Solution 3
You just need to wrap your pattern in a proper delimiter, like forward slashes. Like so:
$pattern = '/\b[A-Z0-9._%+-][email protected][A-Z0-9.-]+\.[A-Z]{2,4}\b/';
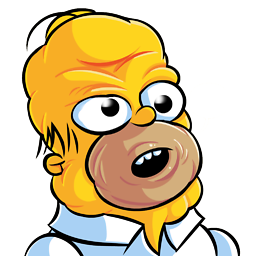
Comments
-
homerun about 2 months
I'm trying to get emails out of a string:
$string = "bla bla [email protected] MIME-Version: [email protected] bla bla bla"; $matches = array(); $pattern = '\b[A-Z0-9._%+-][email protected][A-Z0-9.-]+\.[A-Z]{2,4}\b'; preg_match_all($pattern,$string,$matches); print_r($matches);
The error I'm getting is :
Delimiter must not be alphanumeric or backslash
I got the regex syntax from here http://www.regular-expressions.info/email.html
-
Shridhar over 9 yearsAdd /i or just / after last b( that is in pattern)
-
PeeHaa over 9 yearspossible duplicate of How to validate an email address in PHP
-
-
homerun over 9 yearsive tried it before , $matches comes out empty..
-
Ian McMahon over 9 yearsin that case, your regex needs adjusting. The syntax error is fixed by delimiting properly; constructing a valid regex is a whole different matter :)
-
homerun over 9 yearsive tried it before , $matches comes out empty..
-
Admin over 9 yearsit about your pattern... change play with it...
-
homerun over 9 yearsworks great , can you explain what causes the error and what you did to fix it?
-
Winston over 9 years@MorSela Yes, the first you need had to add delimiters // at start and end of the pattern. After need had to add "i" modificator for case insensitive. That's all. :)
-
homerun over 9 yearsthanks for that m8 , accepted asnwer :)
-
Winston over 9 years@MorSela Thanks :)
-
j5Dev over 9 yearsFor those interested, this may help (Not my script, so not a plug). I swear by it since I found it, it's saved me so much time! - cybernetnews.com/online-regular-expression-builder
-
Winston over 9 years@j5Dev I also use regex builde, this one: regexbuddy.com very cool tools :)
-
homerun over 9 years@Winston hey Winston got 1 more question if you dont mind.. same question here as my main question , just about that regex : [a-z0-9!#$%&'*+/=?^_
{|}~-]+(?:\.[a-z0-9!#$%&'*+/=?^_
{|}~-]+)*@(?:[a-z0-9](?:[a-z0-9-]*[a-z0-9])?\.)+[a-z0-9](?:[a-z0-9-]*[a-z0-9])? (the error:Unknown modifier '=' ) thanks in advance! got that regex as well from that webpage - regular-expressions.info/email.html -
Winston over 9 years@MorSela Do not mind :) Can you show how you're using this pattern in the function (
preg_match
orpreg_match_all
) -
homerun over 9 years@winston preg_match_all , im basically using the same code as the main question , just testing different regex patterns (article author says thats this long regex is the best one so far so locate emails) :)
-
Winston over 9 years@MorSela I've corrected, your the regex pattern #[a-z\d!\#$%&'*+/=?^_
{|}~-]+(?:\.[a-z\d!\#$%&'*+/=?^_
{|}~-]+)*@(?:[a-z\d](?:[a-z\d-]*[a-z\d])?\.)+[a-z\d](?:[a-z\d-]*[a-z\d])?# There is a caveat, for example regex pattern/here your pattern/
, here you're using/
pattern delimiter, therefore in your pattern you must escape each/
char. Also, for example, if you're using#
as pattern delimiter you must escape each#
char in your pattern, etc... -
Winston over 9 yearsYou receive an error because used pattern delimiter in your pattern, without escaping it.
-
homerun over 9 years@Winston works great , thank you so much!!
-
Winston over 9 years@MorSela You're welcome :)
-
ReeCube almost 4 years@Winston thank you for your answer, that's amazing! But now with the new TLD's you should change the 4 into 24.
-
SherylHohman over 2 years@homerrrrrrrr In addition to adding proper delimiters, you would need to add the regex
i
flag, so the email search is case insensitive. The matches came out empty because it was using case sensitive search. The error you got is because the pattern was not properly wrapped in delimiters. The answer above does address the question, which was about an error message. A separate, 2nd, question would need to be asked if you then want to know why you got no matches.