Horizontal Scroll using buttons on angular2
Solution 1
Import ViewChild and ElementRef to get elemenet refrence.
use #localvariable as shown here, <div #widgetsContent class="middle">
get element in component, @ViewChild('widgetsContent', { read: ElementRef }) public widgetsContent: ElementRef<any>;
change scrollvalue, this.widgetsContent.nativeElement.scrollTo({ left: (this.widgetsContent.nativeElement.scrollLeft + 150), behavior: 'smooth' });
An example is shown below
import { Component, OnInit, ViewChild, ElementRef } from "@angular/core";
@Component({
selector: 'my-app',
template: `
<div class="container">
<div style="float: left">
<button (click)="scrollLeft()">left</button>
</div>
<div #widgetsContent class="middle">
<div class="info-widget">
WIDGET
</div>
<div class="info-widget">
WIDGET
</div>
<div class="info-widget">
WIDGET
</div>
<div class="info-widget">
WIDGET
</div>
<div class="info-widget">
WIDGET
</div>
<div class="info-widget">
WIDGET
</div>
</div>
<div style="float: right">
<button (click)="scrollRight()">right</button>
</div>
</div>
`,
styles: [`
.info-widget {
width: 31.75%;
border: 1px solid black;
display: inline-block;
}
.middle {
float: left;
width: 90%;
overflow: auto;
/*will change this to hidden later to deny scolling to user*/
white-space: nowrap;
}
`]
})
export class AppComponent {
@ViewChild('widgetsContent', { read: ElementRef }) public widgetsContent: ElementRef<any>;
public scrollRight(): void {
this.widgetsContent.nativeElement.scrollTo({ left: (this.widgetsContent.nativeElement.scrollLeft + 150), behavior: 'smooth' });
}
public scrollLeft(): void {
this.widgetsContent.nativeElement.scrollTo({ left: (this.widgetsContent.nativeElement.scrollLeft - 150), behavior: 'smooth' });
}
}
Solution 2
Consider the following solution
In .html
<div #widgetsContent class="custom-slider-main">
<div class="info-widget">
WIDGET
</div>
<div class="info-widget">
WIDGET
</div>
<div class="info-widget">
WIDGET
</div>
<div class="info-widget">
WIDGET
</div>
<div class="info-widget">
WIDGET
</div>
<div class="info-widget">
WIDGET
</div>
</div>
In .scss
.custom-slider-main{
display: flex;
overflow: hidden;
scroll-behavior: smooth;
}
In .ts
@ViewChild('widgetsContent') widgetsContent: ElementRef;
And On click of left/right button use the following functions
scrollLeft(){
this.widgetsContent.nativeElement.scrollLeft -= 150;
}
scrollRight(){
this.widgetsContent.nativeElement.scrollLeft += 150;
}
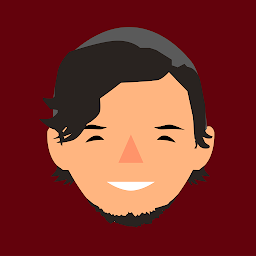
Sergio Mendez
Updated on February 09, 2021Comments
-
Sergio Mendez about 3 years
So I have this app in angular2 where I need to scroll a component horizontally but with buttons right and left. So I need a function for each button that scroll to right or left the content. I need something like this:
I tried using
document.getElementById('myscrolldiv').animate({ scrollLeft: "-=" + 250 + "px"; }
but Angular does not recognize theanimate
line.So I am looking for a diferent way of scroll horizontally using buttons but NOT using jquery. Is there any way to do this in angular?
Here is my html
<div class="container"> <div class="side"> <mat-icon (click)="scrollLeft()">keyboard_arrow_left</mat-icon> </div> <div id="widgetsContent" class="middle"> <div class="scrolls"> <div class="info-widget"> WIDGET </div> <div class="info-widget"> WIDGET </div> <div class="info-widget"> WIDGET </div> <div class="info-widget"> WIDGET </div> <div class="info-widget"> WIDGET </div> <div class="info-widget"> WIDGET </div> </div> </div> <div class="side"> <mat-icon (click)="scrollRight()">keyboard_arrow_right</mat-icon> </div> </div>
And here is my css
.container { display: flex; height: 22.5vh !important; } .side { width: 50px; height: 22.5vh !important; } .middle { flex-grow: 1; height: 22.5vh !important; overflow-x: scroll; overflow-y: hidden; white-space: nowrap; }
So, how do I scroll right and left pushing the buttons? please help.
-
sankar over 5 yearspublic widgetsContent: ElementRef<any> - this does not work in Angular 5. Error: type ElementRef is not generic. When I googled the error, people were pointing at upgrading material version but we are not using material at all in our project.
-
Hari9513 over 5 yearsThanks, this works for me but i want to display scroll based on screen width. "scrolling.this.widgetsContent.nativeElement.scrollLeft + 150" don't want to hard core this 150
-
emre ozcan over 3 years@Hari9513 You can get scrollWidth dynamically.
scrollLeft() { this.widgetsContent.nativeElement.scrollLeft -= this.widgetsContent.nativeElement.scrollWidth; } scrollRight() { this.widgetsContent.nativeElement.scrollLeft += this.widgetsContent.nativeElement.scrollWidth; }