How can I create a Drag and Drop interface?
Solution 1
Tkinter doesn't have any direct support for drag and drop within an application. However, drag and drop requires not much more than making suitable bindings for a button click (<ButtonPress-1>
), the mouse moving while the button is clicked (<B1-Motion>
), and when the button is released (<ButtonRelease-1>
).
Here is a very simplestic example which is designed to work with your code.
First, we'll create a class that can manage the dragging and dropping. It's easier to do this as a class rather than a collection of global functions.
class DragManager():
def add_dragable(self, widget):
widget.bind("<ButtonPress-1>", self.on_start)
widget.bind("<B1-Motion>", self.on_drag)
widget.bind("<ButtonRelease-1>", self.on_drop)
widget.configure(cursor="hand1")
def on_start(self, event):
# you could use this method to create a floating window
# that represents what is being dragged.
pass
def on_drag(self, event):
# you could use this method to move a floating window that
# represents what you're dragging
pass
def on_drop(self, event):
# find the widget under the cursor
x,y = event.widget.winfo_pointerxy()
target = event.widget.winfo_containing(x,y)
try:
target.configure(image=event.widget.cget("image"))
except:
pass
To use it, all you need to do is call the add_draggable
method, giving it the widget(s) you wish to drag.
For example:
label = Label(canvas, image=image)
...
dnd = DragManager()
dnd.add_dragable(label)
...
root.mainloop()
That's all it takes for the basic framework. It's up to you to create a floating draggable window, and to perhaps highlight the item(s) that can be dropped on.
Other implementations
For another implementation of the same concept, see https://github.com/python/cpython/blob/master/Lib/tkinter/dnd.py
Solution 2
https://github.com/akheron/cpython/blob/master/Lib/tkinter/dnd.py
I tested it and it still seems to work in python 3.6.1, I suggest experimenting with it and making it your own, because it doesn't seem to be officially supported in Tkinter.
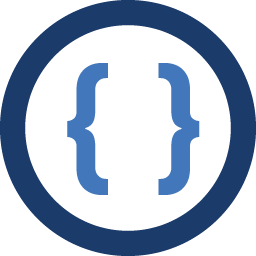
Admin
Updated on May 23, 2021Comments
-
Admin almost 3 years
Currently, I am working with Python 3.5 GUI development using tkinter module. I want to be able to drag an image from one place to another within the application. Does tkinter support drag and drop within an application, and if so, how do you do it?
from tkinter import * root = Tk() root.geometry("640x480") canvas = Canvas(root, height=480, width=640, bg="white") frame = Frame(root, height=480, width=640, bg="white") frame.propagate(0) image = PhotoImage(file="C:/Users/Shivam/Pictures/Paint/Body.png") label = Label(canvas, image=image) label.pack() label_2 = Label(frame, text="Drop Here !") label_2.pack() label_2.place(x=200, y=225, anchor=CENTER) canvas.pack(side=LEFT) frame.pack() root.mainloop()