How can I make a PDF file using base64 string ? Swift
12,838
Yes, you have to convert it to Data and then save it to the documents directory on the device. A function like this would work:
func saveBase64StringToPDF(_ base64String: String) {
guard
var documentsURL = (FileManager.default.urls(for: .documentDirectory, in: .userDomainMask)).last,
let convertedData = Data(base64Encoded: base64String)
else {
//handle error when getting documents URL
return
}
//name your file however you prefer
documentsURL.appendPathComponent("yourFileName.pdf")
do {
try convertedData.write(to: documentsURL)
} catch {
//handle write error here
}
//if you want to get a quick output of where your
//file was saved from the simulator on your machine
//just print the documentsURL and go there in Finder
print(documentsURL)
}
Related videos on Youtube
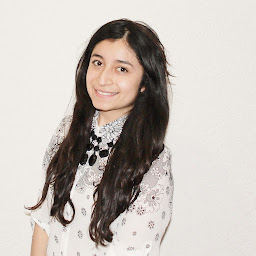
Author by
Elizabeth Hernandez
Updated on June 19, 2022Comments
-
Elizabeth Hernandez almost 2 years
I'm looking for a way to save a PDF file on my project directory, I've received a base 64 pdf string from a Web Service yet. Do I have to convert it to NSData or something like that?
I'm new at coding in Swift but I can follow your instructions.
I hope you can help me. Thanks
-
GetSwifty over 7 yearsDecode the base 64 string into Data, then save the data
-
-
Rahul about 7 yearsthis is working. I tried in swift 3.0
-
Sergio over 4 yearshow can we open the pdf from the app?
-
Anees almost 4 yearshow to display this PDF file later, from document directory in PDFView