Display PDF file in Swift 4
17,401
By seeing your code it seems that you missed to add UIDocumentInteractionControllerDelegate delegate method.
class ViewController: UIViewController,UIDocumentInteractionControllerDelegate {
override func viewDidLoad() {
super.viewDidLoad()
var docController = UIDocumentInteractionController.init(url: URL(fileURLWithPath: NSTemporaryDirectory()).appendingPathComponent(urlVal!))
docController.delegate = self
docController.presentPreview(animated: true)
}
func documentInteractionControllerViewControllerForPreview(_ controller: UIDocumentInteractionController) -> UIViewController {
return self
}
}
OR
You can also view PDF by loading it into WKWebView.
override func viewDidLoad() {
super.viewDidLoad()
let pdfFilePath = Bundle.main.url(forResource: "iostutorial", withExtension: "pdf")
let urlRequest = URLRequest.init(url: pdfFilePath!)
webView = WKWebView(frame: self.view.frame)
webView.load(request)
self.view.addSubview(webView)
}
Related videos on Youtube
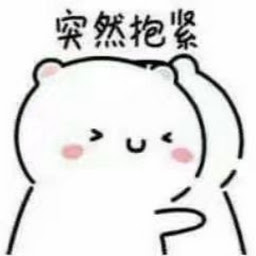
Author by
GTeckHan Goh
Updated on June 04, 2022Comments
-
GTeckHan Goh almost 2 years
import UIKit import WebKit class ViewController: UIViewController, UIDocumentInteractionControllerDelegate { var docController: UIDocumentInteractionController! override func viewDidLoad() { super.viewDidLoad() docController = UIDocumentInteractionController.init(url: URL(fileURLWithPath: NSTemporaryDirectory()).appendingPathComponent(urlVal!)) docController.delegate = self docController.presentPreview(animated: true) } func documentInteractionControllerViewControllerForPreview(_ controller: UIDocumentInteractionController) -> UIViewController { return self } }
Above code I'm not able to display the pdf file. Can anyone help?
-
Abhishek Jain about 6 yearsWhere is your docController defined? I can't see it in the above code.
-
GTeckHan Goh about 6 yearsHi, i define at the top
-
geisterfurz007 about 6 yearsPossible duplicate of Open PDF file using swift
-
-
GTeckHan Goh about 6 years...sorry i miss out to adding here..so sorry.. i got add the func documentInteractionControllerViewControllerForPreview actually
-
GTeckHan Goh about 6 yearscant assign value of type WKWebView to type UIWebView!
-
GTeckHan Goh about 6 yearsi already doing this but the pdf still cant show..the pdf get from one URL from DB
-
Mobile Team iOS-RN about 6 yearsYes you can do that by using this code. override func viewDidLoad() { super.viewDidLoad() let pdfFilePath = Bundle.main.url(forResource: "iostutorial", withExtension: "pdf") let urlRequest = URLRequest.init(url: pdfFilePath!) let webView = UIWebView(frame: self.view.frame) webView.loadRequest(urlRequest) self.view.addSubview(webView) }
-
GTeckHan Goh about 6 yearsi hit error Thread1 : EXC_BAD_INSTRUCTION(code=EXC_1386_INVOP, subcode=0x0) at let urlRequest = URLRequest.init(url: pdfFilePath!)
-
Mobile Team iOS-RN about 6 yearsActually it's working code in my project, All you need to do is just replace your PDF file path.
-
GTeckHan Goh about 6 yearsdunnoe why i hit Thread1 : EXC_BAD_INSTRUCTION(code=EXC_1386_INVOP, subcode=0x0)
-
Apparao Mulpuri about 6 yearsok. Download the PDF file from the URL and pass the file url path to the DocumentInteractionController.
-
GTeckHan Goh about 6 yearsBut..i need to use URL to get the pdf file oh
-
Apparao Mulpuri about 6 yearsright. write the pdf content to a file in your app's Documents folder. Get the file URL from that file.
-
GTeckHan Goh about 6 yearsya..currently i get from DB the URL
-
Apparao Mulpuri about 6 yearsYou have the PDF url but no PDF content. You should download the PDF Content from that URL (URL from DB) and write to the file and pass that file url to DocumentInteractionController.
-
GTeckHan Goh about 6 yearswhich mean..inside my program need have the pdf file ?
-
GTeckHan Goh about 6 years...if i download the pdf and upload to my project..after that i do if let fileURL = Bundle.main.path(forResource: "myPDF", ofType: "pdf")
-
Mobile Team iOS-RN about 6 yearsCan you share me your pdf url?
-
GTeckHan Goh about 6 years
-
Apparao Mulpuri about 6 yearsok. Check this out on how to download and save the file in iOS app bundle. stackoverflow.com/questions/5323427/…
-
Mobile Team iOS-RN about 6 yearsUsing above code i am able to load your PDF, Make sure you enable app transport security key into your plist file.
-
GTeckHan Goh about 6 yearshow to do that ? please guild me
-
GTeckHan Goh about 6 yearshow to do that ? please guild me
-
GTeckHan Goh about 6 yearsIs App Transport Security Setting -> Allow Arbitrary Loads ??
-
Mobile Team iOS-RN about 6 yearsYes add this key value. <key>NSAppTransportSecurity</key> <dict> <key>NSAllowsArbitraryLoads</key> <true/> </dict>