How can I simulate mouse events from code?
Solution 1
You're looking for the SendInput
function, which allows you to synthesize mouse
movements and button clicks in your code by specifying an array of INPUT
structures corresponding to input events.
UINT WINAPI SendInput(
__in UINT nInputs, // number of structures in the pInputs array
__in LPINPUT pInputs, // an array of INPUT structures, representing an event
__in int cbSize // the size, in bytes, of an INPUT structure
);
Note, however, that this function is subject to User Interface Privilege Isolation (UIPI), which means that your application is only permitted to inject input to applications that are running at an equal or lesser integrity level.
Solution 2
Use mouse_event
(winuser.h). The following code will move the mouse then perform a click at the new location. You can do this in two lines but this is more verbose.
Note that X and Y are specified in mickeys, 0 to 65535. This is then mapped onto the current resolution, i.e. 0,0 will be the top left corner and 65535,65535 will be the lower right hand corner.
mouse_event(MOUSEEVENTF_MOVE | MOUSEEVENTF_ABSOLUTE, x, y, 0, 0);
mouse_event(MOUSEEVENTF_LEFTDOWN, 0, 0, 0, 0);
mouse_event(MOUSEEVENTF_LEFTUP, 0, 0, 0, 0);
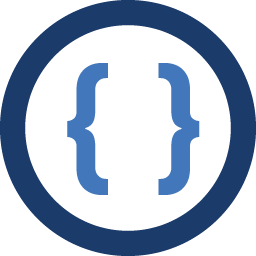
Admin
Updated on July 20, 2022Comments
-
Admin almost 2 years
I would like to simulate mouse events using the Win32 API; how can I do it?
What I want to do is simulate the event at the most basic level, the level at which the system has just the event type and the co-ordinates and hasn't yet figured which window it must relay it to.
I don't know if that's how things work. Either way, I need help doing it. Would I have to meddle at the driver level?!
To make my requirements clear, I don't want to target any window, I just want the system to think the mouse was clicked or moved by the user. And I would be coding in C.
-
Micka over 9 yearsthanks for mentioning the
integrity level
giving my application admin rights solved that problem for me :) -
Winter Dragoness over 9 yearsAccording to MSDN's docs, mouse_event has been superseded by SendInput. They recommend using SendInput instead.
-
jrh over 5 yearsThe mouse_event function doesn't seem to work on Windows 10, Cody's SendInput method does, though.