How do I round up currency values in Java?
Solution 1
I would use something like:
BigDecimal result = new BigDecimal("50.5399999").setScale(2, BigDecimal.ROUND_HALF_UP);
There is a great article called Make cents with BigDecimal on JavaWorld that you should take a look at.
Solution 2
You should use a decimal or currency type to represent money, not floating point.
Solution 3
Math with money is more complex than most engineers think (over generalization)
If you are doing currency calculations, I think you may be delving into problems that seem simple at their surface but are actually quite complex. For instance, rounding methods that are a result of business logic decisions that are repeated often can drastically affect the totals of calculations.
- I would recommend looking at the Java Currency class for currency
formatting. - Also having a look at this page on representing money in java may be helpful.
If this is homework, showing the teacher that you have thought through the real-world problem rather than just slung a bunch of code together that "works" - will surely be more impressive.
On a side note, I initially was going to suggest looking at the implementation of the Java math methods in the source code, so I took a look. I noticed that Java was using native methods for its rounding methods - just like it should.
However, a look at BigDecimal shows that there is Java source available for rounding in Java. So rather than just give you the code for your homework, I suggest that you look at the BigDecimal private method doRound(MathContext mc) in the Java source.
Solution 4
If 50.54 isn't representable in double precision, then rounding won't help.
If you're trying to convert 50.53999999 to a whole number of dollars and a whole number of cents, do the following:
double d = 50.539999; // or however many 9's, it doesn't matter
int dollars = (int)d;
double frac = d - dollars;
int cents = (int)((frac * 100) + 0.5);
Note that the addition of 0.5 in that last step is to round to the nearest whole number of cents. If you always want it to round up, change that to add 0.9999999 instead of 0.5.
Solution 5
Why would you not want to use any Math functions?
static long round(double a)
-Returns the closest long to the argument.
http://java.sun.com/j2se/1.4.2/docs/api/java/lang/Math.html
To represent money I would take the following advice instead of re-inventing the wheel:
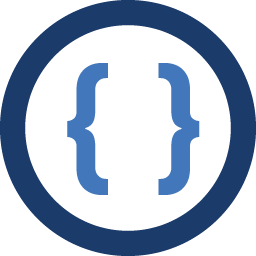
Admin
Updated on September 20, 2020Comments
-
Admin over 3 years
Okay, here's my problem. Basically I have this problem.
I have a number like .53999999. How do I round it up to 54 without using any of the Math functions?
I'm guessing I have to multiply by 100 to scale it, then divide? Something like that?
The issue is with money. let's say I have $50.5399999 I know how to get the $50, but I don't have how to get the cents. I can get the .539999 part, but I don't know how to get it to 54 cents.
-
Admin about 15 yearsThe problem is that we have not covered anyting regarding 'static' and 'round'. The issue is with money. let's say I have $50.5399999 I know how to get the $50, but I don't have how to get the cents. I can get the .539999 part, but I don't know how to get it to 54 cents.
-
Admin about 15 yearsI commented on the first reply on what exactly I am trying to do. "The issue is with money. let's say I have $50.5399999 I know how to get the $50, but I don't have how to get the cents. I can get the .539999 part, but I don't know how to get it to 54 cents."
-
Chris Ballance about 15 yearsI added a note about representing money with Java
-
Sean Bright about 15 yearsI liked your first response better.
-
Chris Ballance about 15 yearsYes, floating point currency storage can lead to very bad things after a bit of computation.
-
strager about 15 yearsAt those who assume "round up" means "always round up" ... This could be the case, yes. Just replace 0.5 in @Ruten's example with 0.9999999... Or, negate and round down, then negate again.
-
Svante about 15 yearsThis is important! Never use floating point numbers for money! Bad things will happen!
-
gnomed about 15 yearsi wish people would comment why they downvote, i dont see how this isnt acceptable code...
-
chburd about 15 yearsBigDecimal is indeed the answer
-
Andrzej Doyle about 15 yearsThe Currency class doesn't actually do any formatting, nor does it store any monetary values; it's just holds a little bit of information about the properties of a currency, of which getDefaultFractionDigits() is the only really relevant one here (and duplicates information in the question anyway).
-
Clayton about 15 years@gnomed: agreed: downvote should get a comment. It looks fine to me, so one up. only improvement would be to package this as a function with the precision passed as a param.
-
Anne Porosoff about 15 yearsStore currency as a long (number of cents)
-
Albert about 15 yearsWhat about fractional cents (calculations involving percents of money may give rise to fractional cents).
-
B Bulfin about 15 years@Albert: Most currency types can handle this. Otherwise use another decimal type, or long number of 1/100 cents.
-
sbilstein about 15 yearsnot sure what you are asking? I changed my answer because the question was edited.