How do I use req.flash() with EJS?
Solution 1
I know this is an old question, but I recently ran across it when trying to understand flash messages and templates myself, so I hope this helps others in my situation. Considering the case of Express 4, the express-flash module, and an ejs template, here are 2 routes and a template that should get you started.
First generate the flash message you want to display. Here the app.all()
method maps to /express-flash
. Request baseurl/express-flash
to create a message using the req.flash(type, message)
setter method before being redirected to baseurl/
.
app.all('/express-flash', req, res ) {
req.flash('success', 'This is a flash message using the express-flash module.');
res.redirect(301, '/');
}
Next map the message to the template in the res.render()
method of the target route, baseurl/
. Here the req.flash(type)
getter method returns the message or messages matching the type, success
, which are mapped to the template variable, expressFlash
.
app.get('/', req, res ) {
res.render('index', {expressFlash: req.flash('success') });
}
Finally, display the value of expressFlash
, if it exists, in index.ejs
.
<p> Express-Flash Demo </p>
<% if ( expressFlash.length > 0 ) { %>
<p>Message: <%= expressFlash %> </p>
<% } %>
Then start the server and visit baseurl/express-flash
. It should trigger a redirect to baseurl/
with the flash message. Now refresh baseurl/
and see the message disappear.
Solution 2
<% if ( message ) { %>
<div class="flash"><%= message %></div>
<% } %>
Is this what you want? You can use some client-side JS to have it fading out. jQuery example:
var message = $( '.message' );
if ( message.length ) {
setTimeout( function() {
message.fadeOut( 'slow' );
}, 5000 );
}
Solution 3
req.flash()
can be used in two ways.
If you use two arguments
req.flash(type, message);
where type
is a type of message and message
is actual message (both strings), then it adds message
to the queue of type type
. Using it with one argument
req.flash(type);
returns array of all messages of type type
and empties the queue. Additionally this method is attached to the session, so it works per session. In other words, each user has its own set of flash queues. So in your view you can do something like this:
var messages = req.flash('info');
and then send the messages
variable to the template and use it there (note that messages
is an array and you can iterate it). Remember that using req.flash('info', 'test');
will append a test
message of type info
only for a current user (associated with the req
object).
Keep in mind that this mechanism is quite weak. For example if a user double clicks on link (two requests send to the server), then he will not see messages, because the first call will empty the queue (of course unless the request generates messages).
Solution 4
I was struggling with this as well; Getting my flash message to appear in my .ejs. BUT, I finally got it to WORK and this is what I understand.
Once the getter req.flash('_msgName_');
is called it is cleared!
Also when you app.use(session());
cookie must equal cookie: {maxAge: 720}
, essentially a big number.
I was using a console.log() to test the getter, and it was displaying in my console, but not in my .ejs. I took the console.log() out and it worked.
Here is some of my code.
Server.js ~
app.get('/', function(req, res) {
// This is where we will retrieve the users from the database and include them in the view page we will be rendering.
User.find({},function(err, allUsers){
if(err){
console.log("Oh no we got an error\n ERROR :: "+err);
} else {
// console.log(allUsers);
res.render('index',{users : allUsers, msg : req.flash('vError')});
}
});
});
// Add User Request
app.post('/users', function(req, res) {
console.log("REQUESTED DATA:\t", req.body);
var user = new User(
{
name: req.body.name,
age: req.body.age
}
);
// Saves user to DB.
user.save(function(err) {
if(err) {
console.log('OOPS, Something went Wrong... \n ERROR :: '+err+'\n');
for(var key in err.errors){
// console.log(err.errors[key].message);
req.flash('vError', err.errors[key].message);
}
// **HERE I WAS ACCIDENTALLY CLEARING IT!!!** \\
// console.log(req.flash('vError'));
// res.render('index',{users : [], msg : req.flash('vError')});
res.redirect('/');
} else {
console.log('\tSuccessfully added a new User to the DB!');
res.redirect('/');
}
})
});
index.ejs ~
<% if(msg.length > 0) { %>
<% for (var error in msg) { %>
<h3><%= msg[error] %></h3>
<% } %>
<% } %>
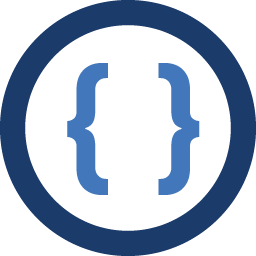
Admin
Updated on July 09, 2022Comments
-
Admin almost 2 years
I want to be able to flash a message to the client with Express and EJS. I've looked all over and I still can't find an example or tutorial. Could someone tell me the easiest way to flash a message?
Thanks!
-
Admin almost 12 yearsThis still doesn't tell me how I display it in a template (EJS). I already know how
req.flash()
works. -
freakish almost 12 yearsSo you don't know how to use templating engine? Then why did you ask about
req.flash()
? This has nothing to do withflash
then. Do you know how to pass and use variables in EJS? -
Admin almost 12 yearsI never said that. I mean, how do I display it in my view? And yeah, been using Express + EJS for ages now. Just never had to use flash messages. It does have something to do with flash, I want to know how I display the flash message in my view.
-
freakish almost 12 yearsAnd how is that different from displaying normal array of strings??
-
Admin almost 12 yearsI don't know, why don't you tell me since I don't have any idea what variable(s) get passed to the view. If I knew that, I wouldn't have posted this question.
-
Jamie Chapman over 10 yearsSorry to comment on on an old post, but express-messages now only works with Express 2.x only.
-
Poison Oak about 9 yearsFor a more complete explanation, I created a Gist including an informative readme about flash messaging in Express 4. It includes two approaches to flash messaging, one using the express-session module alone, and the other using the express-flash module. It also includes templates in ejs, handlebars, and jade.
-
nacho4d almost 9 yearsThanks @Poison Oak after hours I just realised I was missing the
=
in<%=
! that is why my messages were always empty!