how to add button click event in android studio
Solution 1
SetOnClickListener (Android.View.view.OnClickListener) in View cannot be applied to (com.helloandroidstudio.MainActivity)
This means in other words (due to your current scenario) that your MainActivity need to implement OnClickListener:
public class Main extends ActionBarActivity implements View.OnClickListener {
// do your stuff
}
This:
buttonname.setOnClickListener(this);
means that you want to assign listener for your Button "on this instance" ->
this instance represents OnClickListener and for this reason your class have to implement that interface.
It's similar with anonymous listener class (that you can also use):
buttonname.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View view) {
}
});
Solution 2
Button button= (Button)findViewById(R.id.buttonId);
button.setOnClickListener(new View.OnClickListener(){
@Override
public void onClick(View view) {
// click handling code
}
});
Solution 3
package com.mani.smsdetect;
import android.app.Activity;
import android.os.Bundle;
import android.view.View;
import android.widget.Button;
public class MainActivity extends Activity implements View.OnClickListener {
//Declaration Button
Button btnClickMe;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
//Intialization Button
btnClickMe = (Button) findViewById(R.id.btnClickMe);
btnClickMe.setOnClickListener(MainActivity.this);
//Here MainActivity.this is a Current Class Reference (context)
}
@Override
public void onClick(View v) {
//Your Logic
}
}
Solution 4
package com.mani.helloworldapplication;
import android.app.Activity;
import android.os.Bundle;
import android.support.design.widget.FloatingActionButton;
import android.support.design.widget.Snackbar;
import android.support.v7.app.AppCompatActivity;
import android.support.v7.widget.Toolbar;
import android.view.View;
import android.view.Menu;
import android.view.MenuItem;
import android.widget.Button;
import android.widget.TextView;
import android.widget.Toast;
public class MainActivity extends Activity implements View.OnClickListener {
//Declaration
TextView tvName;
Button btnShow;
@Override
protected void onCreate(Bundle savedInstanceState) {
//Empty Window
super.onCreate(savedInstanceState);
//Load XML File
setContentView(R.layout.activity_main);
//Intilization
tvName = (TextView) findViewById(R.id.tvName);
btnShow = (Button) findViewById(R.id.btnShow);
btnShow.setOnClickListener(this);
}
@Override
public void onClick(View v)
{
String name = tvName.getText().toString();
Toast.makeText(MainActivity.this,name,Toast.LENGTH_SHORT).show();
}
}
Solution 5
When you define an OnClickListener
(or any listener) this way
btnClick.setOnClickListener(this);
you need to implement
the OnClickListener
in your Activity
.
public class MainActivity extends ActionBarActivity implements OnClickListener{
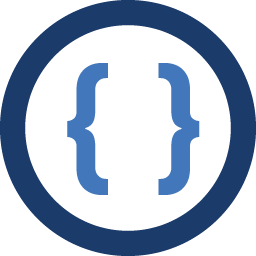
Admin
Updated on July 23, 2021Comments
-
Admin almost 3 years
So I have done some research, and after defining you button as an object by the code
private Button buttonname; buttonname = (Button) findViewById(R.id.buttonnameinandroid) ;
here is my problem
buttonname.setOnClickListener(this); //as I understand it, the "**this**" denotes the current `view(focus)` in the android program
then your
OnClick()
event...Problem:
When I type in the "this", it says:
setOnClickListener (Android.View.view.OnClickListener) in View cannot be applied to (com.helloandroidstudio.MainActivity)
I have no idea why?
here is the code from the .java file
import android.widget.Button; import android.widget.EditText; import android.widget.TextView; public class MainActivity extends ActionBarActivity { private Button btnClick; private EditText Name, Date; private TextView msg, NameOut, DateOut; @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.main); btnClick = (Button) findViewById(R.id.button) ; btnClick.setOnClickListener(this); Name = (EditText) findViewById(R.id.textenter) ; Date = (EditText) findViewById(R.id.editText) ; msg = (TextView) findViewById(R.id.txtviewOut) ; NameOut = (TextView) findViewById(R.id.txtoutName) ; DateOut = (TextView) findViewById(R.id.txtOutDate) ; if (savedInstanceState == null) { getSupportFragmentManager().beginTransaction() .add(R.id.container, new PlaceholderFragment()) .commit(); } } public void onClick(View v) { if (v == btnClick) { if (Name.equals("") == false && Date.equals("") == false) { NameOut = Name; DateOut = Date; msg.setVisibility(View.VISIBLE); } else { msg.setText("Please complete both fields"); msg.setVisibility(View.VISIBLE); } } return; }