How to allow only English letters in input fields?
Solution 1
I would try this onChange
function:
onChange={(e) => {
let value = e.target.value
value = value.replace(/[^A-Za-z]/ig, '')
this.setState({
value,
})
}}
See the codepen: https://codepen.io/bozdoz/pen/vzJgQB
The idea is to reverse your regex matcher with ^
and replace all non-A-z characters with ''
Solution 2
You can use the pattern attribute in your input.
<input pattern = “[A-Za-z]”>
Solution 3
You can try to use the Regx to solve this issue. Just check the character that entered is an alphabet using regx. If not then don't update the state value.
import React from "react";
class InputValidationDemo extends React.Component {
constructor(props) {
super(props);
this.state = {
type: "text",
name: "username",
value: ""
};
}
onChange = (e) => {
const re = /^[A-Za-z]+$/;
if (e.target.value === "" || re.test(e.target.value))
this.setState({ value: e.target.value });
};
render() {
const { type, name, value } = this.state;
return (
<div>
<input type={type} name={name} value={value} onChange={this.onChange} />
</div>
);
}
}
export default InputValidationDemo;
https://codesandbox.io/s/react-input-only-letters-qyf2j
The important part is the regular expression. You can change your validation type by changing the regex.
Solution 4
This should do the trick, all characters except ascii 0-127 which are English characters should be excluded, o through 127 also gives you space, +, -, / and punctuation which is useful, if you want only letters then [^A-z] should do the trick, if you need non-space characters then [^A-z\s] should work:
document.getElementById('english').addEventListener('input', function(){
this.value = this.value.replace(/[^\x00-\x7F]+/ig, '');
});
React Way:
class InputEnglish extends React.Component {
constructor(){
super();
this.state = {value: ''};
this.onChange = this.onChange.bind(this);
}
onChange(e) {
let val = e.target.value.replace(/[^\x00-\x7F]/ig, '');
this.setState(state => ({ value: val }));
}
render() {
return (<input
value={this.state.value}
onChange={this.onChange}
/>);
}
}
https://codepen.io/anon/pen/QVMgrd
Solution 5
You could use /[A-Za-z]/
regular expression and an input
event handler which is fired every time the value of the element changes. Something like below:
const regex = /[A-Za-z]/;
function validate(e) {
const chars = e.target.value.split('');
const char = chars.pop();
if (!regex.test(char)) {
e.target.value = chars.join('');
console.log(`${char} is not a valid character.`);
}
}
document.querySelector('#myInput').addEventListener('input', validate);
<label for="myInput">Type some text:</label>
<input id="myInput" type="text" />
Related videos on Youtube
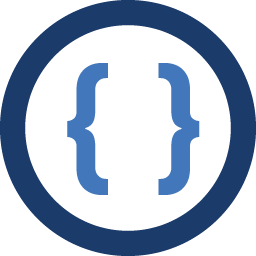
Admin
Updated on August 17, 2021Comments
-
Admin over 2 years
So, this is my input field:
<input type={type} name={name} />
How can I allow only English letters?
This is the
RegEx
, I believe I should use:/[A-Za-z]/ig
https://regex101.com/r/upWFNy/1
I am assuming that
onChange()
event should be used for this with the combination ofsetState()
andevent.target.value
.Thanks.
PS. I need to have this WHILE typing.
-
Kevin Lewis over 5 yearsIt is already outlined here: stackoverflow.com/questions/7144167/…
-
Admin over 5 years@KevinLewis, hi. Can you update your answer, but only for English, no numbers please? Thanks.
-
Tom Blodget over 5 yearsEnglish is an interesting language because it uses many letters are that aren't in its "alphabet". So, are you sure you want this? If so, perhaps you should restate it as upper and lowercase Basic Latin letters.
-
Tom Blodget over 5 yearsNote: You can't tell a web browser what characters to allow or not allow. But you can use JavaScript or HTML patterns that are triggered upon various events to remove unwanted characters. This is what you'll see in the answers.
-
-
Admin over 5 yearsHi, can you write this in React way please?
-
Harry Chilinguerian over 5 years@JohnSam don't do much in React but this should work.
-
Harry Chilinguerian over 5 years@JohnSam take a look at the codepen in the post it works in there. If it's still not working let me know. : )
-
Admin over 5 yearsYes.. but for me the issue is that
value
is always empty. It fills dynamically as I enter the text. That's why it fails atvalue={this.state.value}
@bozdoz -
Admin over 5 yearsYes.. but for me the issue is that
value
is always empty. It fills dynamically as I enter the text. That's why it fails at fetching thevalue
. -
Danziger over 3 yearsThis won't work if I type, paste or drop invalid characters in the middle of the input. Check this out: stackoverflow.com/questions/51065370/…
-
Tom O. over 3 years@Danziger I tried pasting a non-letter character example on Chrome v 85 and it seems to work as I expect. Do you have an example of this not working in a specific browser?
-
Danziger over 3 yearsIt works fine if you paste it at the end. Try moving the cursor to the left and pasting it there. I'm on Chrome 85 as well.
-
George Ogden over 3 yearsFor jQuery, use
$(this).val($(this).val().replace(/[^A-Za-z]/ig, ''))
-
Lexib0y about 3 yearsPlease provide some explanation with your answer.