Validating multiple fields in a form
Solution 1
Your brace should be after function validateForm() and after the if, and at the end of the function. Overall, the braces are screwed in this example.
Lay your code out so the opening and closing braces match up and make sense to you.
Solution 2
Fixed code: jsfiddle
<!DOCTYPE html>
<html>
<head>
<script type="text/javascript">
function validateForm() {
var x=document.forms["myForm"]["name"].value;
if (x==null || x=="")
{
alert("Name must be filled out");
return false;
}
var y=document.forms["myForm"]["password"].value;
if (y==null || y=="") {
alert("Password name must be filled out");
return false;
}
}
</script>
</head>
<body>
<form name="myForm" action="demo_form.asp" onsubmit="return validateForm()" method="post">
Name*: <input type="text" name="name"> <br>
Password*: <input type="password" name="password"><br>
Email: <input type="text" name="email"><br>
<input type="submit" value="Submit">
</form>
</body>
<html>
Be careful of where you place your braces. Additionally, it is advantageous to use the console on your browser to identify some errors and fixed them.
Solution 3
You missed some braces {}
and one was in the wrong spot.
Hope this works:
function validateForm() {
var x=document.forms["myForm"]["name"].value;
if (x==null || x=="")
{
alert("Name must be filled out");
return false;
}
var y=document.forms["myForm"]["password"].value;
{
if (y==null || y=="")
alert("Password name must be filled out");
return false;
}
}
Solution 4
You misplaced the braces { }
for validation of password. Place them after if
clause.
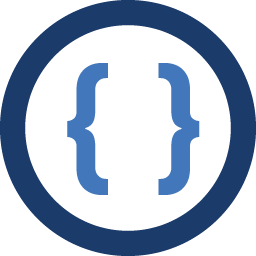
Admin
Updated on December 16, 2020Comments
-
Admin over 3 years
I was doing the testing for the first time. I read the this code and made one of my own from it. The thing is that its not giving any error even if the fields are left empty.
Here is my fiddle.
Please help out. Thanks.
<!DOCTYPE html> <html> <head> <script type="text/javascript"> {function validateForm() var x=document.forms["myForm"]["name"].value; if (x==null || x=="") { alert("Name must be filled out"); return false; } var y=document.forms["myForm"]["password"].value; { if (y==null || y=="") alert("Password name must be filled out"); return false; } </script> </head> <body> <form name="myForm" action="demo_form.asp" onsubmit="return validateForm()" method="post"> Name*: <input type="text" name="name"> <br> Password*: <input type="password" name="password"><br> Email: <input type="text" name="email"><br> <input type="submit" value="Submit"> </form> </body> </html>
-
compski over 5 yearsHi Giorgos, your link is broken, can you upload this in jsfiddle or something?