How to call constructors in main()?
Apart from many other syntax errors in the code you have provided, constructing objects with automatic storage duration should look like this:
class A {
public:
A() { } // <-- default constructor
A(int i) { } // <-- constructor taking int
void foo() { } // <-- member function ("method")
static s() { } // <-- static function
};
and somewhere:
A a; // <-- constructs object using the default constructor
A a2 = A(); // <-- equivalent to the previous one (copy initialization)
A a3(a2); // <-- copy constructor ~ constructs a3 using the a2 object
A a4(7); // <-- constructs object using the constructor taking int
// now when you have some objects, you can call member functions
// (i.e. invoke the behavior they provide)
a.foo();
// static functions are not dependent on objects:
A::s(); // <-- calls static function defined within namespace of class A
Before you continue in writing more codes, consider spending some time reading some decent book.
In case you're not sure about what book to read, here you might find one: The Definitive C++ Book Guide and List
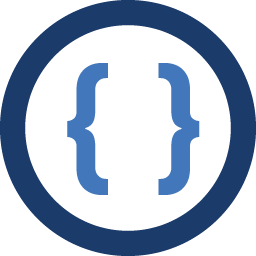
Admin
Updated on July 09, 2022Comments
-
Admin almost 2 years
I'm working on a program that takes a
string
and aint
as variables for myfriend
constructors. I have most of my program done, but since I'm new to this topic offriend
s and constructors with C++, I don't know how to implement the call on mymain()
to use these parameters and start working on myistream
andostream
to ask the user to input the values and start printing it.Could someone please guide me the right way?
These are my current constructors:
jellyBeanFlavors::jellyBeanFlavors() { flavor = "null"; jellyBeans = 0; } jellyBeanFlavors::jellyBeanFlavors(int newJellyBeans) { flavor = "null"; jellyBeans = newJellyBeans; } jellyBeanFlavors::jellyBeanFlavors(string newFlavor, int newjellyBeans) { flavor = newFlavor; jellyBeans = newjellyBeans; } void jellyBeanFlavors::output() { cout << flavor << " " << jellyBeans << endl; }
Now I'm trying to implement here my objects and start making my questions for input and then print with my
istream
function:int main () { jellyBeanFlavors::jellyBeanFlavors(string newFlavor, int newjellyBeans); jellyBeanFlavors(); jellyBeanFlavors myFirstFlavor = jellyBeanFlavors.the; jellyBeanFlavors mySecondFlavor; jellyBeanFlavors total = 0; cout << "Your first flavor is: "<< myFirstFlavor. << endl; cin >> myFirstFlavor; cout << "Your second flavor is: "<< mySecondFlavor << endl; cin >> mySecondFlavor; cout << "The expected result of adding" << " the first flavor loaded with" << mySecondFlavor <<" in the quantity with jellybean2 loaded with 6 in the quantity is: "; cout << "a jellybean with a quantity of 11. cout << "the result of adding jellybean1 and jellybean two is: " << jellybean1 + jellybean2 << endl; // this isnt implemented right but i need advice please on how to call my objects from my main class. system("pause"); return 0; }
so you dont get confused here is my main class:
#include <iostream> #include <string> using namespace std; class jellyBeanFlavors { friend jellyBeanFlavors operator + (jellyBeanFlavors theFirstJellyBean, jellyBeanFlavors theSecondJellyBean);//sums friend jellyBeanFlavors operator - (jellyBeanFlavors theFirstJellyBean, jellyBeanFlavors theSecondJellyBean);//(binary) substracts friend jellyBeanFlavors operator - (jellyBeanFlavors theFirstJellyBean);// (unary) checks negatives friend jellyBeanFlavors operator * (jellyBeanFlavors theFirstJellyBean,jellyBeanFlavors theSecondJellyBean);//multiplies friend jellyBeanFlavors operator / (jellyBeanFlavors theFirstJellyBean,jellyBeanFlavors theSecondJellyBean);//divides friend jellyBeanFlavors operator == (jellyBeanFlavors theFirstJellyBean,jellyBeanFlavors theSecondJellyBean);//compares friend jellyBeanFlavors operator < (jellyBeanFlavors theFirstJellyBean,jellyBeanFlavors theSecondJellyBean);// less than friend jellyBeanFlavors operator > (jellyBeanFlavors theFirstJellyBean,jellyBeanFlavors theSecondJellyBean);//greater than friend ostream& operator << (ostream& outputStream, const jellyBeanFlavors& jellyBeanOutput);// output friend istream& operator >> (istream& inputStream, jellyBeanFlavors& jellyBeanInput);//input public: jellyBeanFlavors(); jellyBeanFlavors(int); jellyBeanFlavors(string, int); void output(); private: string flavor; int jellyBeans; };
> my solution to my program by adding objects and overloading them:
my solution to my program by adding objects and overloading them:
int main () { system("cls"); char op; jellyBeanFlavors obj1,obj2,obj3; do{ cin>>obj1; //flavor cin>>obj2; //amount system("cls"); cout<<"JELLYBEANS:"<<endl; obj3=obj1+obj2; cout<<"\n The Sum is : "; obj3.output(); obj3=obj1-obj2; cout<<"\n The Substraction is : "; obj3.output(); obj3=obj1*obj2; cout<<"\n The Multiplication is: "; obj3.output(); obj3=obj1/obj2; cout<<"\n The Divide operation is : "; obj3.output(); cout<<"\n"; obj3 = obj1==obj2; obj3.output(); cout<<"\n"; obj3 = obj1>obj2; obj3.output(); cout<<"\n"; obj3 = obj1<obj2; obj3.output(); cout<<"\n"; obj3 = -obj1; obj3.output(); cout<<"\n"; obj3 = -obj2; obj3.output(); cout<<"\n"; cout<<"\n\nPress A/a and Enter to continue or 0 to exit"<<endl; cin>>op; if(op = 0) { exit(0); } }while(op =='a'|| op=='A'); system("pause"); }