How to control defaultValue of React Native's <TextInput>?
15,790
Solution 1
For one, the render
method can't return multiple nodes. I believe the <TextInput/>
should be wrapped by the <TouchableHighlight/>
component, like:
render() {
return (
<TouchableHighlight onPress={this.sendOff}>
<TextInput
defaultValue='PassMeIn'
onChange={this.changeName}
value={this.state.nameNow}
/>
</TouchableHighlight>
)
}
This will make it possible to pass a prop through from <TouchableHightlight>
to <TextInput
to use as the defaultValue
and you'll have access to it in the <TouchableHighlight>
component.
Solution 2
You can store the defaultValue inside a new state and then you can access it in both places
constructor(props) {
super(props);
this.state = {
//...other state
defaultValue: "PassMeIn"
};
//...other code
sendOff() {
//...do things with this.state.defaultValue
}
render() {
return (
<TextInput
defaultValue={this.state.defaultValue}
onChange={this.changeName}
value={this.state.nameNow}
/>
<TouchableHighlight onPress={this.sendOff}/>
)
}
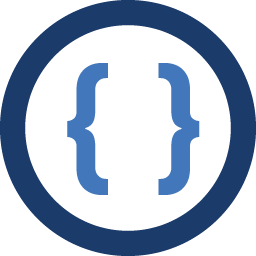
Author by
Admin
Updated on July 19, 2022Comments
-
Admin almost 2 years
I have a
<TextInput/>
with adefaultValue
set up and a button where it would trigger and pass thedefaultValue
of<TextInput>
elsewhere.But how do I pass in the
<TextInput/>
'sdefaultValue
to theonPress
method of<TouchableHighlight/>
?This is what I am trying to achieve:
changeName(text) { this.setState({nameNow: text}) //How can I immediately set the defaultValue='PassMeIn' to nameNow? Because this method would never be called unless text is changed within TextInput } sendOff() { //Would like to access the defaultValue='PassMeIn' here whenever sendOff() is called. How can I? } render() { return ( <View> <TextInput defaultValue='PassMeIn' onChange={this.changeName} value={this.state.nameNow} /> <TouchableHighlight onPress={this.sendOff}/> <Text>Button</Text> </TouchableHighlight> </View> ) }