How to divide an array by an other array element wise in numpy?
12,968
It is simple to do in pure numpy, you can use broadcasting to calculate the outer product (or any other outer operation) of two vectors:
import numpy as np
a = np.arange(1, 4)
b = np.arange(1, 4)
c = a[:,np.newaxis] / b
# array([[1. , 0.5 , 0.33333333],
# [2. , 1. , 0.66666667],
# [3. , 1.5 , 1. ]])
This works, since a[:,np.newaxis]
increases the dimension of the (3,)
shaped array a
into a (3, 1)
shaped array, which can be used for the desired broadcasting operation.
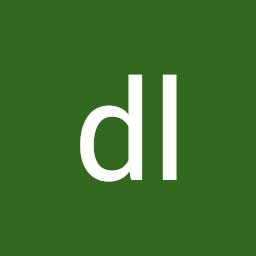
Author by
dl wu
Updated on June 04, 2022Comments
-
dl wu almost 2 years
I have two arrays, and I want all the elements of one to be divided by the second. For example,
In [24]: a = np.array([1,2,3]) In [25]: b = np.array([1,2,3]) In [26]: a/b Out[26]: array([1., 1., 1.]) In [27]: 1/b Out[27]: array([1. , 0.5 , 0.33333333])
This is not the answer I want, the output I want is like (we can see all of the elements of a are divided by b)
In [28]: c = [] In [29]: for i in a: ...: c.append(i/b) ...: In [30]: c Out[30]: [array([1. , 0.5 , 0.33333333]), array([2. , 1. , 0.66666667]), In [34]: np.array(c) Out[34]: array([[1. , 0.5 , 0.33333333], [2. , 1. , 0.66666667], [3. , 1.5 , 1. ]])
But I don't like for loop, it's too slow for big data, so is there a function that included in numpy package or any good (faster) way to solve this problem?