How to import and use a custom font in a material-ui theme?
Solution 1
Instead of installing via npm, you can just first load CSS file.
@import url('https://fonts.googleapis.com/css?family=Yellowtail&display=swap');
Import this CSS file
import './assets/css/yellowtail.css';
Now you don't need to use any @font-face. This can be used with font families like normal.
Solution 2
You are missing three things:
- Import the npm package as something
- Use the
CssBaseline
component - Add an
override
to the object provided tocreateMuiTheme()
See example:
import React, { Component, Fragment } from 'react';
import Header from './Components/Layouts/Header';
import AppBody from './Components/Layouts/AppBody';
import Footer from './Components/Layouts/Footer';
import { MuiThemeProvider, createMuiTheme, CssBaseline } from '@material-ui/core';
import yellowtail from 'typeface-yellowtail';
const theme = createMuiTheme({
typography: {
fontFamily:
'"Yellowtail", cursive',
},
overrides: {
MuiCssBaseline: {
'@global': {
'@font-face': [yellowtail],
},
},
},
});
class App extends Component {
render() {
return (
<MuiThemeProvider theme={theme}>
<CssBaseline />
<Header />
<AppBody />
<Footer />
</MuiThemeProvider>
);
}
}
export default App;
Example CodeSandbox (MUI v3): https://codesandbox.io/s/late-pond-gqql4?file=/index.js
Example CodeSandbox (MUI v4): https://codesandbox.io/s/pensive-monad-eqwlx?file=/index.js
Notes
MuiThemeProvider
changed toThemeProvider
in the transition from Material-UI v3 to v4. If you are on v4, this is the only change needed - this example code otherwise works on both versions.You must wrap text in Material-UI's
Typography
component for the font to be used.
Solution 3
Update: Since @ekkis mentioned I'm adding further details on how to include the Google Font. The following answer assumes you're using Material UI v4.10.1
Install the gatsby-plugin-web-font-loader to install Google font.
Edit
gatsby-config.js
to include the plugin and the Google font.
module.exports = {
plugins: [
{
resolve: "gatsby-plugin-web-font-loader",
options: {
google: {
families: ["Domine", "Work Sans", "Happy Monkey", "Merriweather", "Open Sans", "Lato", "Montserrat"]
}
}
},
]
}
- To use the font, create a file called
theme.js
insrc/components
import {
createMuiTheme,
responsiveFontSizes
} from "@material-ui/core/styles"
let theme = createMuiTheme({
typography: {
fontFamily: [
"Work Sans",
"serif"
].join(","),
fontSize: 18,
}
})
// To use responsive font sizes, include the following line
theme = responsiveFontSizes(theme)
export default theme
Now that you've the
theme
export, you can use it in your components.Import
theme
on to your components. Let's say<Layout />
is your main component.
// src/components/layout.js
/**
* External
*/
import React from "react"
import PropTypes from "prop-types"
import {
ThemeProvider
} from "@material-ui/styles"
import { Typography } from "@material-ui/core"
/**
* Internal
*/
import theme from "./theme"
const Layout = ({ children }) => {
return (
<>
<ThemeProvider theme={theme}>
<Typography variant="body1">Hello World!</Typography>
</ThemeProvider>
</>
)
}
- To use Google font in other components, just use
<Typography ...>Some Text</Typography>
As long as these components use as their parent, these components will continue to use the Google Font. Hope this helps.
Old Answer:
Place your text in the Typography
component to reflect the Google Font you installed via npm
import { Typography } from "@material-ui/core"
Assume you have some text in <AppBody>
<AppBody>
Hello World!
</AppBody>
The above text should now be changed to
<AppBody>
<Typography variant="body1">Hello World!</Typography>
</AppBody>
Refer Material UI docs for the different variants.
Solution 4
There is a much easier way of doing this that doesn't require a .css
file or installing any extra packages.
If your font is available over a CDN like Google Fonts then just imported into the root HTML file of your app:
<link
href="https://fonts.googleapis.com/css2?family=Yellowtail&display=swap"
rel="stylesheet"
/>
Then add one line in the Material-UI theme:
const theme = createTheme({
typography: { fontFamily: ["Yellowtail", "cursive"].join(",") }
});
Related videos on Youtube
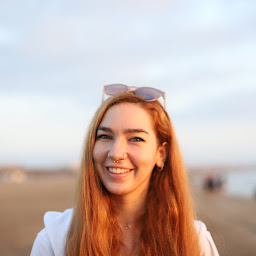
boglarkaszell
Updated on September 15, 2022Comments
-
boglarkaszell over 1 year
I'm trying to import and use the Yellowtail font (from Google Fonts) in my React app in a Material-UI theme.
As far as i know all google fonts are on npm, I've installed it, with the
npm install typeface-yellowtail --save
command.
I have imported it in App.js, put it in the font-family part of the theme, passed the theme to the MuiThemeProvider, but it does not work. What did I miss?
This is what i have inside of App.js (header contains an AppBar with some grids and body contains only an h1 text for testing)
import React, { Component, Fragment } from 'react'; import Header from './Components/Layouts/Header'; import AppBody from './Components/Layouts/AppBody'; import Footer from './Components/Layouts/Footer'; import { MuiThemeProvider, createMuiTheme } from '@material-ui/core'; import 'typeface-yellowtail'; const theme = createMuiTheme({ typography: { fontFamily: '"Yellowtail", cursive', }, }); class App extends Component { render() { return ( <MuiThemeProvider theme={theme}> <Header /> <AppBody /> <Footer /> </MuiThemeProvider> ); } } export default App;
-
boglarkaszell over 4 yearsThank you for your help! I wanted to avoid this approach, hence i'd like to work with the style settings provided by material-ui.
-
ekkis almost 4 yearsyour code doesn't work. where is CssBaseLine defined? and what kind of font has no extension (i.e.
typeface-yellowtail
)? andThemeProvider
doesn't exist in v4 butMuiThemeProvider
does -
ekkis almost 4 yearsand where does
yellowtail.css
come from and what does it contain? -
psychedelicious almost 4 years@ekkis I believe you are mistaken about
ThemeProvider
... See the v4 documentation here: material-ui.com/customization/theming And see the v3 documentation here: material-ui.com/customization/theming -
psychedelicious almost 4 years@ekkis I somehow didn't get the import for
CssBaseline
in the answer, I have edited it. It is a component provided by Material-UI: material-ui.com/api/css-baseline/#cssbaseline-apitypeface-yellowtail
is an NPM package, not a font file. The OP has installed it usingnpm install typeface-yellowtail --save
, per the question. -
ekkis almost 4 yearsthank you for the additions, which help for those who haven't done this before. much better!
-
Megajin over 3 yearsCareful! If the client has a slow internet connection, the loaded font will take a while. This method requires a separate HTTP request to Googles CDN. Until the request is completed, the fonts will fallback to a standard font.
-
BackSlashHaine about 3 yearsAnd how did you do if its a premium purchased .otf files without any CDN ?
-
Joel Rummel over 2 yearsThis is vastly easier than many of the other answers on the web that involve installation of packages.