How to insert multiple documents at once in MongoDB through Java
Solution 1
DBCollection.insert
accepts a parameter of type DBObject
, List<DBObject>
or an array of DBObject
s for inserting multiple documents at once. You are passing in a string array.
You must manually populate documents(DBObject
s), insert them to a List<DBObject>
or an array of DBObject
s and eventually insert
them.
DBObject document1 = new BasicDBObject();
document1.put("name", "Kiran");
document1.put("age", 20);
DBObject document2 = new BasicDBObject();
document2.put("name", "John");
List<DBObject> documents = new ArrayList<>();
documents.add(document1);
documents.add(document2);
collection.insert(documents);
The above snippet is essentially the same as the command you would issue in the MongoDB shell:
db.people.insert( [ {name: "Kiran", age: 20}, {name: "John"} ]);
Solution 2
Before 3.0, you can use below code in Java
DB db = mongoClient.getDB("yourDB");
DBCollection coll = db.getCollection("yourCollection");
BulkWriteOperation builder = coll.initializeUnorderedBulkOperation();
for(DBObject doc :yourList)
{
builder.insert(doc);
}
BulkWriteResult result = builder.execute();
return result.isAcknowledged();
If you are using mongodb version 3.0 , you can use
MongoDatabase database = mongoClient.getDatabase("yourDB");
MongoCollection<Document> collection = database.getCollection("yourCollection");
collection.insertMany(yourDocumentList);
Solution 3
As of MongoDB 2.6 and 2.12 version of the driver you can also now do a bulk insert operation. In Java you could use the BulkWriteOperation. An example use of this could be:
DBCollection coll = db.getCollection("user");
BulkWriteOperation bulk = coll.initializeUnorderedBulkOperation();
bulk.find(new BasicDBObject("z", 1)).upsert().update(new BasicDBObject("$inc", new BasicDBObject("y", -1)));
bulk.find(new BasicDBObject("z", 1)).upsert().update(new BasicDBObject("$inc", new BasicDBObject("y", -1)));
bulk.execute();
Solution 4
Creating Documents
There're two principal commands for creating documents in MongoDB:
insertOne()
insertMany()
There're other ways as well such as Update
commands. We call these operations, upserts. Upserts occurs when there're no documents that match the selector used to identify documents.
Although MongoDB inserts ID by it's own, We can manually insert custom IDs as well by specifying _id
parameter in the insert...()
functions.
To insert multiple documents we can use insertMany()
- which takes an array of documents as parameter. When executed, it returns multiple id
s for each document in the array. To drop the collection, use drop()
command. Sometimes, when doing bulk inserts - we may insert duplicate values. Specifically, if we try to insert duplicate _id
s, we'll get the duplicate key error
:
db.startup.insertMany( [ {_id:"id1", name:"Uber"}, {_id:"id2", name:"Airbnb"}, {_id:"id1", name:"Uber"}, ] );
MongoDB stops inserting operation, if it encounters an error, to supress that - we can supply ordered:false
parameter. Ex:
db.startup.insertMany( [ {_id:"id1", name:"Uber"}, {_id:"id2", name:"Airbnb"}, {_id:"id1", name:"Airbnb"}, ], {ordered: false} );
Solution 5
Your insert record format like in MongoDB that query retire from any source EG.
{
"_id" : 1,
"name" : a
}
{
"_id" : 2,
"name" : b,
}
it is mongodb 3.0
FindIterable<Document> resulutlist = collection.find(query);
List docList = new ArrayList();
for (Document document : resulutlist) {
docList.add(document);
}
if(!docList.isEmpty()){
collectionCube.insertMany(docList);
}
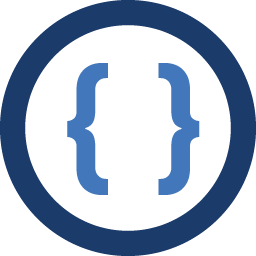
Admin
Updated on July 20, 2020Comments
-
Admin almost 4 years
I am using MongoDB in my application and was needed to insert multiple documents inside a MongoDB collection . The version I am using is of 1.6
I saw an example here
http://docs.mongodb.org/manual/core/create/
in the
Bulk Insert Multiple Documents Section
Where the author was passing an array to do this .
When I tried the same , but why it isn't allowing , and please tell me how can I insert multiple documents at once ??
package com; import java.util.Date; import com.mongodb.BasicDBObject; import com.mongodb.DB; import com.mongodb.DBCollection; import com.mongodb.MongoClient; public class App { public static void main(String[] args) { try { MongoClient mongo = new MongoClient("localhost", 27017); DB db = mongo.getDB("at"); DBCollection collection = db.getCollection("people"); /* * BasicDBObject document = new BasicDBObject(); * document.put("name", "mkyong"); document.put("age", 30); * document.put("createdDate", new Date()); table.insert(document); */ String[] myStringArray = new String[] { "a", "b", "c" }; collection.insert(myStringArray); // Compilation error at this line saying that "The method insert(DBObject...) in the type DBCollection is not applicable for the arguments (String[])" } catch (Exception e) { e.printStackTrace(); } } }
Please let me know what is the way so that I can insert multiple documents at once through java .