How to make one side circular border of widget with flutter?
92,663
Solution 1
Use BorderRadius.only
and provide the sides
return Center(
child: Container(
height: 100,
width: 100,
decoration: BoxDecoration(
borderRadius: BorderRadius.only(
topRight: Radius.circular(40),
),
border: Border.all(
width: 3,
color: Colors.green,
style: BorderStyle.solid,
),
),
child: Center(
child: Text(
"Hello",
),
),
),
);
Output
Solution 2
You can achieve this by following code for creating your widget :
return Container(
width: 150.0,
padding: const EdgeInsets.all(20.0),
decoration: BoxDecoration(
shape: BoxShape.rectangle,
color: Colors.white,
borderRadius: BorderRadius.only(
topLeft: Radius.circular(20.0),
topRight: Radius.zero,
bottomLeft: Radius.zero,
bottomRight: Radius.zero,
),
),
child: Text(
"hello",
),
);
This way you can have your top left sided circular border with Container widget in flutter.
Solution 3
If you want one side of a container rounded you want to use BorderRadius.only
and specify which corners to round like this:
Container(
width: 150.0,
padding: const EdgeInsets.all(20.0),
decoration: BoxDecoration(
borderRadius: BorderRadius.only(
topRight: Radius.circular(40.0),
bottomRight: Radius.circular(40.0)),
color: Colors.white),
child: Text("hello"),
),
Solution 4
Another way of doing this is to use the ClipRRect widget. Simply wrap your widget with ClipRRect and give a radius. You can specify which corner you want to make round.
ClipRRect(
borderRadius: BorderRadius.only(topRight: Radius.circular(10)),
child: Container(
height: 40,
width: 40,
color: Colors.amber,
child: Text('Hello World!'),
),
);
Solution 5
also do can do as follows,
borderRadius: new BorderRadius.only(
topLeft: const Radius.circular(30.0),
bottomLeft: const Radius.circular(30.0),
),
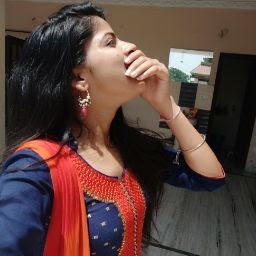
Author by
Shruti Ramnandan sharma
Updated on July 05, 2022Comments
-
Shruti Ramnandan sharma almost 2 years
I'm trying to build one side circular border with Container widget in flutter. I have searched for it but can't get any solution.
Container( width: 150.0, padding: const EdgeInsets.all(20.0), decoration: BoxDecoration( // borderRadius: BorderRadius.circular(30.0), /* border: Border( left: BorderSide() ),*/ color: Colors.white ), child: Text("hello"), ),