How to read a selected text file from sdcard on android
24,536
Try this code:
package com.javasamples;
import java.io.*;
import android.app.Activity;
import android.os.Bundle;
import android.view.*;
import android.view.View.OnClickListener;
import android.widget.*;
public class FileDemo2 extends Activity {
// GUI controls
EditText txtData;
Button btnWriteSDFile;
Button btnReadSDFile;
Button btnClearScreen;
Button btnClose;
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.main);
// bind GUI elements with local controls
txtData = (EditText) findViewById(R.id.txtData);
txtData.setHint("Enter some lines of data here...");
btnWriteSDFile = (Button) findViewById(R.id.btnWriteSDFile);
btnWriteSDFile.setOnClickListener(new OnClickListener() {
public void onClick(View v) {
// write on SD card file data in the text box
try {
File myFile = new File("/sdcard/mysdfile.txt");
myFile.createNewFile();
FileOutputStream fOut = new FileOutputStream(myFile);
OutputStreamWriter myOutWriter =
new OutputStreamWriter(fOut);
myOutWriter.append(txtData.getText());
myOutWriter.close();
fOut.close();
Toast.makeText(getBaseContext(),
"Done writing SD 'mysdfile.txt'",
Toast.LENGTH_SHORT).show();
} catch (Exception e) {
Toast.makeText(getBaseContext(), e.getMessage(),
Toast.LENGTH_SHORT).show();
}
}// onClick
}); // btnWriteSDFile
btnReadSDFile = (Button) findViewById(R.id.btnReadSDFile);
btnReadSDFile.setOnClickListener(new OnClickListener() {
public void onClick(View v) {
// write on SD card file data in the text box
try {
File myFile = new File("/sdcard/mysdfile.txt");
FileInputStream fIn = new FileInputStream(myFile);
BufferedReader myReader = new BufferedReader(
new InputStreamReader(fIn));
String aDataRow = "";
String aBuffer = "";
while ((aDataRow = myReader.readLine()) != null) {
aBuffer += aDataRow + "\n";
}
txtData.setText(aBuffer);
myReader.close();
Toast.makeText(getBaseContext(),
"Done reading SD 'mysdfile.txt'",
Toast.LENGTH_SHORT).show();
} catch (Exception e) {
Toast.makeText(getBaseContext(), e.getMessage(),
Toast.LENGTH_SHORT).show();
}
}// onClick
}); // btnReadSDFile
btnClearScreen = (Button) findViewById(R.id.btnClearScreen);
btnClearScreen.setOnClickListener(new OnClickListener() {
public void onClick(View v) {
// clear text box
txtData.setText("");
}
}); // btnClearScreen
btnClose = (Button) findViewById(R.id.btnClose);
btnClose.setOnClickListener(new OnClickListener() {
public void onClick(View v) {
// clear text box
finish();
}
}); // btnClose
}// onCreate
}// AndSDcard
the layout file is
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout
android:id="@+id/widget28"
android:layout_width="fill_parent"
android:layout_height="fill_parent"
android:background="#ff0000ff"
android:orientation="vertical"
xmlns:android="http://schemas.android.com/apk/res/android"
>
<EditText
android:id="@+id/txtData"
android:layout_width="fill_parent"
android:layout_height="180px"
android:textSize="18sp" />
<Button
android:id="@+id/btnWriteSDFile"
android:layout_width="143px"
android:layout_height="44px"
android:text="1. Write SD File" />
<Button
android:id="@+id/btnClearScreen"
android:layout_width="141px"
android:layout_height="42px"
android:text="2. Clear Screen" />
<Button
android:id="@+id/btnReadSDFile"
android:layout_width="140px"
android:layout_height="42px"
android:text="3. Read SD File" />
<Button
android:id="@+id/btnClose"
android:layout_width="141px"
android:layout_height="43px"
android:text="4. Close" />
</LinearLayout>
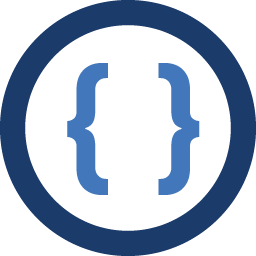
Author by
Admin
Updated on June 29, 2020Comments
-
Admin almost 4 years
i am new at android development and i need your help. I was locking at topics that are similar for my development but non of then help me. So far i create functions that gets me the files from my sdcard and shows me the list of then. That is working
this is the code for getting the paths on sdcard:
package com.seminarskirad; import android.app.AlertDialog; import android.app.AlertDialog.Builder; import android.app.ListActivity; import android.content.Context; import android.content.DialogInterface; import android.content.Intent; import android.net.Uri; import android.os.Bundle; import android.os.Environment; import android.provider.MediaStore; import android.util.Log; import android.view.View; import android.view.View.OnClickListener; import android.widget.ArrayAdapter; import android.widget.ListView; import android.widget.TextView; import java.io.BufferedReader; import java.io.File; import java.io.FileNotFoundException; import java.io.FileReader; import java.io.FilenameFilter; import java.io.IOException; import java.net.URISyntaxException; import java.util.ArrayList; import java.util.List; public class LoadActivity extends ListActivity{ private enum DISPLAYMODE{ ABSOLUTE, RELATIVE; } private final DISPLAYMODE displayMode = DISPLAYMODE.ABSOLUTE; private List<String> directoryEntries = new ArrayList<String>(); private File currentDirectory = new File("/"); @Override public void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); Browse(Environment.getExternalStorageDirectory()); } private void upOneLevel(){ if(this.currentDirectory.getParent() != null) this.Browse(this.currentDirectory.getParentFile()); } private void Browse(final File aDirectory){ if (aDirectory.isDirectory()){ this.currentDirectory = aDirectory; fill(aDirectory.listFiles()); } } private void fill(File[] files) { this.directoryEntries.clear(); if(this.currentDirectory.getParent() != null) this.directoryEntries.add(".."); switch(this.displayMode){ case ABSOLUTE: for (File file : files){ this.directoryEntries.add(file.getPath()); } break; case RELATIVE: // On relative Mode, we have to add the current-path to the beginning int currentPathStringLenght = this.currentDirectory.getAbsolutePath().length(); for (File file : files){ this.directoryEntries.add(file.getAbsolutePath().substring(currentPathStringLenght)); } break; } ArrayAdapter<String> directoryList = new ArrayAdapter<String>(this, R.layout.load, this.directoryEntries); this.setListAdapter(directoryList); } protected void onListItemClick(ListView l, View v, int position, long id) { int selectionRowID = position; String selectedFileString = this.directoryEntries.get(selectionRowID); if(selectedFileString.equals("..")){ this.upOneLevel(); }else if(selectedFileString.equals()){ /// what to write here ??? this.readFile(); ///what to write here??? } else { File clickedFile = null; switch(this.displayMode){ case RELATIVE: clickedFile = new File(this.currentDirectory.getAbsolutePath() + this.directoryEntries.get(selectionRowID)); break; case ABSOLUTE: clickedFile = new File(this.directoryEntries.get(selectionRowID)); break; } if(clickedFile.isFile()) this.Browse(clickedFile); } } private void readFile() { // what to write here??? }
Sorry i cant put the image because i dont have reputation, but when i run it on my emulator a get something like this:
/mnt/sdcard/kuzmanic.c /mnt/sdcard/text.txt /mnt/sdcard/DCIM /mnt/sdcard/LOST.DIR
So what I want to do is when i click on the text.txt or kuzmanic.c file I want to open then in the same layout file, that is my load.xml file:
This is the code for the xml file: <?xml version="1.0" encoding="utf-8"?> <TextView xmlns:android="http://schemas.android.com/apk/res/android" android:layout_width="fill_parent" android:layout_height="fill_parent" android:textSize="18sp"> </TextView>
What i need to write in my code and do I have to write anything in the manifest???