How to read and overwrite text file in C?
Solution 1
long pos = ftell(fp);//Save the current position
while (fgets(buffer, 500, fp) != NULL) {
buffer[0] = 'x';
fseek(fp, pos, SEEK_SET);//move to beginning of line
fprintf(fp, "%s", buffer);
fflush(fp);
pos = ftell(fp);//Save the current position
}
Solution 2
I always suggest to use another file do this kindda solutions.
- Read the line
- Put x in a new file in a line and the copy the rest of the line.
- Do this till you get EOF
- remove the old file
- rename this new file
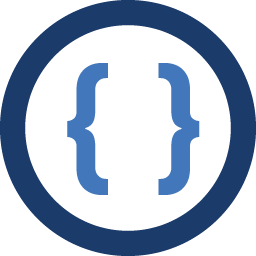
Admin
Updated on June 19, 2022Comments
-
Admin almost 2 years
I have a text file text.txt that reads (for simplicity purposes)
this is line one this is line two this is line three
Again for simplicity's sake, I am just trying to set the first character in each line to 'x', so my desired result would be
xhis is line one xhis is line two xhis is line three
So I am opening the text.txt file and trying to overwrite each line with the desired output to the same text file. In the while loop, I set the first character in each line to 'x'. I also set the variable "line" equal to one, because if its on the first line, I want to rewind to the beginning of the file in order to overwrite at the start instead of at the end of the file. Line is then incremented so it will skip the rewind for the next iteration, and should continue to overwrite the 2nd and 3rd lines. It works perfectly for the first line.
Anybody have any solutions? BTW, I have researched this extensively both on stackoverflow and other sites, and no luck. Here's my code and my output is also below:
#include <stdio.h> #include <stdlib.h> #define MAX 500 int main() { char *buffer = malloc(sizeof(char) * MAX); FILE *fp = fopen("text.txt", "r+"); int line = 1; while (fgets(buffer, 500, fp) != NULL) { buffer[0] = 'x'; if (line == 1) { rewind(fp); fprintf(fp, "%s", buffer); } else { fprintf(fp, "%s", buffer); } line++; } free(buffer); fclose(fp); }
Output:
xhis is line one this is line two xhis is line two e x
-
Lepidopteron almost 7 yearsAlthough it may seem obvious, what certain code fragments are used to, please consider using code comments or documentation references, when answering a question, so people get additional background information.
-
BLUEPIXY over 6 years@NattachaiSuteerapongpan See 7.21.5.3 The fopen function p7 of n1570.pdf
-
Xiao over 6 yearsI know you your comment was replied 2 years ago but I've just read your answer and I'm not really clear about "fflush()". Your code work absolutely fine but then I have tried remove "fflush(fp)" and run the code. The program stuck in infinite loop. Since I broke the program, it produce very weird result. I have really no idea why result look like. Could you please help me explain why removing fflush produce a result like this. Here is the link of text file(before and after execute program). drive.google.com/drive/folders/…
-
BLUEPIXY over 6 years@NattachaiSuteerapongpan It is necessary to call
fflush
as written in the link shown in the previous comment. It does not always work correctly if it does not exist. Briefly explained, it is buffered, so it needs to be reflected in the actual file. -
Xiao over 6 years@ BLUEPIXY Thanks a lot for your reply:) I've got a lot of ideas from your link mentioned above.