Return FILE pointer from function and work on it on main
10,256
Solution 1
Without all the code I can't explain the warning, but when you "return 1" from the function in the error case you didn't initialize the pointer correctly.
Change to this:
#include <stdio.h>
#include <stdlib.h>
FILE *create_file()
{
FILE *regularTxt = NULL;
regularTxt = fopen("MyLogExamplekkggggggk.txt", "wt");
if (regularTxt) {
char first_part_string[] = "kkkkik";
fprintf(regularTxt, "%s\n\n\n%s", "ttttg", "lklf");
return regularTxt;
}
return NULL; // error
}
int main(void)
{
FILE* p_txt = create_file();
if (p_txt == NULL)
{
printf("error with file");
getchar();
exit(1); // quit
}
fprintf(p_txt, "%s\n\n\n%s", "gggg", "lklf");
return 0;
}
Solution 2
You seem to be treating warnings as errors here. I think you should first check the returned value for NULL
to silence this error:
FILE *p_txt = create_file();
if (! p_txt) {
fprintf(stderr, "[!] Failed to open the file!\n");
return 1;
} else {
fprintf(p_txt, "%s\n\n\n%s", "gggg", "lklf");
}
Also, when you're entering into if (!regularTxt)
, you're returning one from a function returning a pointer. Better return NULL
instead.
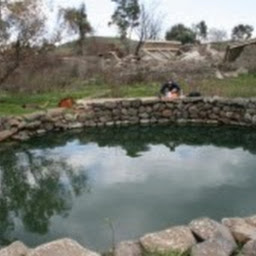
Author by
mikel bubi
Updated on June 17, 2022Comments
-
mikel bubi almost 2 years
I've tried to return a
FILE
pointer from some function tomain()
. After it, I've tried to do somefprintf
on the pointer but it wasn't working. Here is my code:My function:
FILE *create_file(void){ FILE *regularTxt = NULL; regularTxt = fopen("MyLogExamplekkggggggk.txt", "wt"); if (!regularTxt){ printf("error with regtxt"); getchar(); return 1; } char first_part_string[] = "kkkkik"; fprintf(regularTxt, "%s\n\n\n%s", "ttttg", "lklf"); return regularTxt; }
The
main
function:int main(void) { p_txt = create_file(); fprintf(p_txt, "%s\n\n\n%s", "gggg", "lklf"); return 0; }
The error:
Error 92 error C4703: potentially uninitialized local pointer variable 'p_txt' used
-
Andrew Henle almost 8 years
nullptr
is C++, not C. -
Brad almost 8 years@AndrewHenle Correct, fixed. (Habit)
-
Jonathan Leffler almost 8 yearsInterestingly, in
create_file()
, you could move thereturn regularTxt;
out of theif
statement and use it in place of thereturn NULL;
— simply return its value, NULL or not, but don't use it unless it is non-null. -
Brad almost 8 years@JonathanLeffler - True but my goal was to transform the code as directly from the original as possible to make it easier to follow for OP.
-
Jonathan Leffler almost 8 yearsWhat's with the
1 == 2
comparison?