Write pointer to file in C
Solution 1
You need to dereference your pointer and write the parts of the struct. (You should not fwrite a struct directly, but rather encode its parts and write those:
Student *s = malloc(sizeof(*s));
s->name = "Jon";
s->surname = "Skeet";
s->age = 34;
// ....
fwrite(s->name, sizeof(char), strlen(s->name) + 1, fp);
fwrite(s->surname, sizeof(char), strlen(s->surname) + 1, fp);
//This one is a bit dangerous, but you get the idea.
fwrite(&(s->age), sizeof(s->age), 1, fp);
Solution 2
You will have to do some extra work to write out the data pointed to by the structure elements - probably just write it out element by element.
Alternatively change your structure to something like this:
typedef struct
{
char name[MAX_NAME_LEN];
char surname[MAX_SURNAME_LEN];
int age;
} Student;
Solution 3
if you want to directly write the structure as it is to a file you would want to define the sizes of name and surname rather than dynamically allocating it.
typedef structure student { char name[100]; char surname[100]; int age; }
else you will need to write each info in the structure one by one.
Solution 4
In C, you have to serialize structures (convert them to a series of bytes) manually. If you want the data you output to be readable by another machine, you have to take endianness account endianness.
Here's a simple example of serializing your structure (writing it to a file), disregarding endianness/differing word size and making the code unportable:
size_t length;
length = strlen(s->name) + 1;
fwrite(&length, sizeof(length), 1, fp);
fwrite(s->name, 1, length, fp);
length = strlen(s->surname) + 1;
fwrite(&length, sizeof(length), 1, fp);
fwrite(s->surname, 1, length, fp);
fwrite(&s->age, sizeof(s->age), 1, fp);
And to unserialize:
size_t length;
fread(&length, sizeof(length), 1, fp);
s->name = malloc(length);
fread(s->name, 1, length, fp);
fread(&length, sizeof(length), 1, fp);
s->surname = malloc(length);
fread(s->surname, 1, length, fp);
fread(&s->age, sizeof(s->age), 1, fp);
In a real application, you should check the validity of your input rather than blindly assuming the input is valid and trustworthy. Also, you need to decide what byte order you'll use to store your int
s, size_t
s, etc, and make sure you read/write them in the correct byte order by using bit shifting.
By the way, you may want to look at tpl, a simple binary serialization library for C.
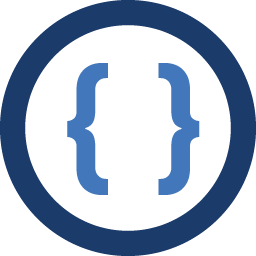
Admin
Updated on June 18, 2022Comments
-
Admin almost 2 years
I have a structure:
typedef struct student { char *name; char *surname; int age; } Student;
I need to write the structure into a binary file.
Here is my attempt :Student *s = malloc(sizeof(*s));
I fill my structure with data and then I write the struct into the file with :
fwrite(s, sizeof(*s), 1, fp);
In my file, name and surname don't exist. Instead they have their respective address of their
char *
pointer.
How can I write achar *
into a file instead of the address of the pointer ?