How to read/process command line arguments?
Solution 1
The canonical solution in the standard library is argparse
(docs):
Here is an example:
from argparse import ArgumentParser
parser = ArgumentParser()
parser.add_argument("-f", "--file", dest="filename",
help="write report to FILE", metavar="FILE")
parser.add_argument("-q", "--quiet",
action="store_false", dest="verbose", default=True,
help="don't print status messages to stdout")
args = parser.parse_args()
argparse
supports (among other things):
- Multiple options in any order.
- Short and long options.
- Default values.
- Generation of a usage help message.
Solution 2
import sys
print("\n".join(sys.argv))
sys.argv
is a list that contains all the arguments passed to the script on the command line. sys.argv[0]
is the script name.
Basically,
import sys
print(sys.argv[1:])
Solution 3
Just going around evangelizing for argparse which is better for these reasons.. essentially:
(copied from the link)
argparse module can handle positional and optional arguments, while optparse can handle only optional arguments
argparse isn’t dogmatic about what your command line interface should look like - options like -file or /file are supported, as are required options. Optparse refuses to support these features, preferring purity over practicality
argparse produces more informative usage messages, including command-line usage determined from your arguments, and help messages for both positional and optional arguments. The optparse module requires you to write your own usage string, and has no way to display help for positional arguments.
argparse supports action that consume a variable number of command-line args, while optparse requires that the exact number of arguments (e.g. 1, 2, or 3) be known in advance
argparse supports parsers that dispatch to sub-commands, while optparse requires setting
allow_interspersed_args
and doing the parser dispatch manually
And my personal favorite:
- argparse allows the type and
action parameters to
add_argument()
to be specified with simple callables, while optparse requires hacking class attributes likeSTORE_ACTIONS
orCHECK_METHODS
to get proper argument checking
Solution 4
There is also argparse
stdlib module (an "impovement" on stdlib's optparse
module). Example from the introduction to argparse:
# script.py
import argparse
if __name__ == '__main__':
parser = argparse.ArgumentParser()
parser.add_argument(
'integers', metavar='int', type=int, choices=range(10),
nargs='+', help='an integer in the range 0..9')
parser.add_argument(
'--sum', dest='accumulate', action='store_const', const=sum,
default=max, help='sum the integers (default: find the max)')
args = parser.parse_args()
print(args.accumulate(args.integers))
Usage:
$ script.py 1 2 3 4
4
$ script.py --sum 1 2 3 4
10
Solution 5
If you need something fast and not very flexible
main.py:
import sys
first_name = sys.argv[1]
last_name = sys.argv[2]
print("Hello " + first_name + " " + last_name)
Then run python main.py James Smith
to produce the following output:
Hello James Smith
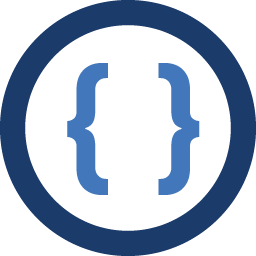
Admin
Updated on March 10, 2021Comments
-
Admin about 3 years
I am originally a C programmer. I have seen numerous tricks and "hacks" to read many different arguments.
What are some of the ways Python programmers can do this?
Related
-
Admin almost 15 yearsAre these built in modules the best? Or can you think of a better custom way?
-
Barry Wark almost 15 yearsYes, these are the best. Since they're part of the standard library, you can be sure they'll be available and they're easy to use. optparse in particular is powerful and easy.
-
user1066101 almost 15 years@edgerA: What does "better custom way" mean?
-
jemfinch about 14 yearsoptparse is one of the best; getopt is old and really ought to be considered deprecated.
-
jpswain over 13 yearsThis is now part of standard Python as of 2.7 and 3.2 :)
-
Xiong Chiamiov over 13 yearsFor really simple stuff, this is the way to go, although you probably only want to use
sys.argv[1:]
(avoids the script name). -
Roberto Bonvallet over 12 yearsThere is already another command-line parsing module named Commando: github.com/lakshmivyas/commando. It wraps argparse by using decorators.
-
oob over 12 yearsat this point (12/2011), argparse is now considered a better option than optparse, correct?
-
earthmeLon almost 12 yearsPython Documentation suggests the use of argparse instead of optparse.
-
ArtOfWarfare over 9 yearsWhat are "optional arguments"? You say they're in optparse. I thought that they were arguments that may or may not be provided, but you said they're in optparse while going on to say that "optparse requires that the exact number of arguments be known in advance". So either your definition of "optional argument" differs from what I thought, or your answer is inconsistent with itself.
-
gvoysey about 8 yearsThis has rapidly become my favorite way to go. It's string parsing so it's kind of brittle, but it's brittle all in one place and you can preview your logic at try.docopt.org . Optional and mutually-exclusive arguments are done in a really elegant way.
-
osman about 7 yearsJust a gripe: argparse documentation is also insanely, insanely complicated. You can't get a simple answer for "how do I make a command line argument take in a single value, and how do I access that value." </gripe>
-
John Lawrence Aspden almost 7 yearsI am desperate to see the rest of the code for naval_fate.py
-
lifebalance almost 7 years@osman This gentle tutorial on argparse might help...
-
Juanjo Salvador almost 7 yearsThis is the best solution. Using
argh
is easier than another libs or usingsys
. -
tripleee over 6 yearsA more realistic usage would be
python main.py "James Smith"
which putsJames Smith
insys.argv[1]
and produces anIndexError
when you try to use the nonexistentsys.argv[2]
. Quoting behavior will somewhat depend on which platform and shell you run Python from. -
tripleee over 6 yearsI wanted to like
argh
but it's not particularly suitable for scenarios where your utmost desire is not to have a command with subcommands. -
Kent Munthe Caspersen over 6 yearsI don't agree that my usage is less realistic. Pretend your program needs to know the exact first and last name of a person to run the script in a business where people can have multiple first and last names? If James Smith has Joseph as an extra first or last name, how would distinguish between whether Joseph is an extra first or last name if you only do
python main.py "James Joseph Smith"
? If you are concerned with index out of bounds, you can add a check for the number of provided arguments. Less realistic or not, my example shows how to handle multiple arguments. -
circular-ruin about 6 years@tripleee YMMV, but I found that this was more of a defect in the documentation than in the library itself. It seems perfectly feasible to have
def frobnicate_spleches(...)
defining a function that does whatever your script does, then doingif __name__ == '__main__': argh.dispatch_command(frobnicate_spleches)
at the end of the file. -
Derek about 6 yearspython and wheel re-invention
-
wim almost 6 yearsSince
optparse
is deprecated, the asker of the question is no longer a member on stack overflow, and this is the accepted answer on a highly visible question - please consider completely rewriting your example code to use stdlibargparse
instead. -
blitu12345 almost 6 yearsits just a copy and paste
-
jfs almost 6 years@blitu12345 at the time of the publication of my answer there were no other answers that mention argparse in any way. The module itself was not in stdlib¶ What do you have against code examples from the documentation? Why do you think it is necessary to come up with your own examples instead of examples provided by the author of the module? And I don't like link-only answers (I'm not alone).
-
blitu12345 almost 6 yearsPeoples coming here already had an idea whats in the documentation and will be here only for further clearance about the topic.Same was my case but what i really found here is a copy and paste from the original docs.Peace!
-
mtraceur almost 6 years@ArtOfWarfare "optional arguments" in this context presumably means arguments specified with option-like arguments such as
-f
or--foo
, while "exact number of arguments be known in advance" presumably means positional arguments given without any preceding option flags. -
sjas over 5 years"Peoples coming here already had an idea whats in the documentation" - i highly doubt that assumtion. somehow.
-
WinEunuuchs2Unix almost 5 yearsAll the other answers are for plotting a lunar landing mission. I'm just simply using
gmail-trash-msg.py MessageID
. This answer is straight forward to testMessageID
parameter has been passed insys.argv[1]
. -
r.mirzojonov over 4 years@AymanHourieh you did not show how to get those arguments. The documentation of this module is a mess.