How to send data to servlet using ajax without a submitting form
46,675
Solution 1
you could either use $.ajax() or $.post here. since you have used $.ajax(). please refer below correction:
<!DOCTYPE html>
<html lang="en">
<head>
<title>SO question 4112686</title>
<script src="http://code.jquery.com/jquery-latest.min.js"></script>
<script>
$(document).ready(function() {
$('#somebutton').click(function() {
$.get('GetUserServlet', function(responseText) {
$('#somediv').text(responseText);
});
});
});
$("#somebutton").click(function(){
$.ajax({
url:'GetUserServlet',
data:{name:'abc'},
type:'get',
cache:false,
success:function(data){
alert(data);
$('#somediv').text(responseText);
},
error:function(){
alert('error');
}
}
);
}
);
</script>
</head>
<body>
<button id="somebutton">press here</button>
<div id="somediv"> </div>
</body>
and your servlet should be:
import java.io.IOException;
import java.io.PrintWriter;
import javax.servlet.*;
import javax.servlet.http.*;
public class GetUserServlet extends HttpServlet {
public void doGet(HttpServletRequest request, HttpServletResponse response)
throws ServletException, IOException {
doPost(request, response);
}
public void doPost(HttpServletRequest request, HttpServletResponse response)
throws ServletException, IOException {
String text = "Update successfull"; //message you will recieve
String name = request.getParameter("name");
PrintWriter out = response.getWriter();
out.println(name + " " + text);
}
Solution 2
You may use $.post method for this purpose.
Here is my solution
index.jsp
<!DOCTYPE html><html lang="en">
<head>
<title>SO question 4112686</title>
<script src="http://code.jquery.com/jquery-latest.min.js"></script>
<script>
$(document).ready(function() {
$("#somebutton").click(function() {
servletCall();
});
});
function servletCall() {
$.post(
"GetUserServlet",
{name : "Message from jsp"}, //meaasge you want to send
function(result) {
$('#somediv').html('Here is your result : <strong>' + result + '</strong>'); //message you want to show
});
};
</script>
</head>
<body>
<button id="somebutton">press here</button>
<div id="somediv"></div>
</body>
</html>
GetUserServlet.java
import java.io.IOException;
import java.io.PrintWriter;
import javax.servlet.*;
import javax.servlet.http.*;
public class GetUserServlet extends HttpServlet {
public void doGet(HttpServletRequest request, HttpServletResponse response)
throws ServletException, IOException {
doPost(request, response);
}
public void doPost(HttpServletRequest request, HttpServletResponse response)
throws ServletException, IOException {
String text = "<br>Message from servlet<br>"; //message you will recieve
String name = request.getParameter("name");
PrintWriter out = response.getWriter();
out.println(text + name);
}
}
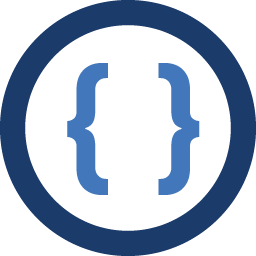
Author by
Admin
Updated on July 23, 2022Comments
-
Admin almost 2 years
I am new with servlet, I am able to get data from the servlet but not able to send data to it and I want to do this without using a submitting form, can i get some help please
on the click of the button it will go to the servlet and return the text but not the value send to it
This is my index.jsp
<!DOCTYPE html> <html lang="en"> <head> <title>SO question 4112686</title> <script src="http://code.jquery.com/jquery-latest.min.js"></script> <script> $(document).ready(function() { $('#somebutton').click(function() { $.get('GetUserServlet', function(responseText) { $('#somediv').text(responseText); }); }); }); $("#somebutton").click(function(){ $.ajax ( { url:'GetUserServlet', data:{name:'abc'}, type:'get', cache:false, success:function(data){alert(data);}, error:function(){alert('error');} } ); } ); </script> </head> <body> <button id="somebutton" onclick="showHint('GetUserServlet.java', 'travis');">press here</button> <div id="somediv"></div> </body>
this my servlet
protected void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException { String text = "Update Sucessful"; String name = request.getParameter("name"); response.setContentType("text/plain"); // Set content type of the response so that jQuery knows what it can expect. response.setCharacterEncoding("UTF-8"); // You want world domination, huh? response.getWriter().write( name + text); // Write response body.