How to set a Modal popup with an image in React
26,692
Try this sample code. Here is a link to the example ImageComponent https://codesandbox.io/s/4xnxqz0ylx
export default class ImageComponent extends React.Component {
state = { isOpen: false };
handleShowDialog = () => {
this.setState({ isOpen: !this.state.isOpen });
console.log('cliked');
};
render() {
return (
<div>
<img
className="small"
src="/Anj.png"
onClick={this.handleShowDialog}
alt="no image"
/>
{this.state.isOpen && (
<dialog
className="dialog"
style={{ position: 'absolute' }}
open
onClick={this.handleShowDialog}
>
<img
className="image"
src="/Anj.png"
onClick={this.handleShowDialog}
alt="no image"
/>
</dialog>
)}
</div>
);
}
}
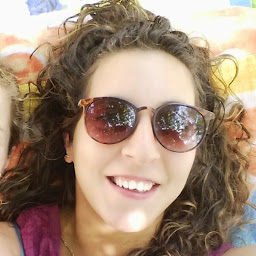
Author by
ShiGi
Updated on July 09, 2022Comments
-
ShiGi almost 2 years
I have an Image class in React, and I have a button that should implement expand on click, on every image. I decided to use a Modal popup so when I click, the Image will show bigger in the Modal popup. I find it hard to set the Modal as an image.
Thank you in advance.
This is from the
Image
class in React:<FontAwesome className="image-icon" name="expand" title="expand" onClick={this.showModal} /> <Modal show={this.state.isExpand} handleClose={this.hideModal} />
Modal:
const Modal = ({ handleClose, show }) => { const showHideClassName = show ? 'modal display-block' : 'modal display-none'; return ( <div className={showHideClassName}> <section className="modal-main"> <button onClick={handleClose}>close</button> </section> </div> ); };