How to take inputs from <Input/> in react semantic UI
Solution 1
Since you have used the arrow
operator, this.inputtext.value
won't work,
you need to write:
sendMessage(){
console.log(this.inputtext.value);
}
In this case semantic-ui's Input
Component is a div
wrapped on top of input
. So you cannot access input element directly through ref. You should use the react's preferred way to get the value, which is
<Input onChange={this.handleMessage.bind(this)} placeholder='message'/>
handleMessage (e) { console.log(e.target.value); }
or without using bind, babel-preset-stage-2
is required for this.
<Input onChange={this.handleMessage} placeholder='message'/>
handleMessage = e => { console.log(e.target.value); }
Solution 2
In your case, you can also get the input value through this code:
this.inputtext.inputRef.value
Solution 3
You need to use a normal class method for this to work. You also shouldn't have a semi-colon in the ref.
sendMessage() {
console.log(this.inputtext.value);
}
render(){
return(
<div>
<Input ref={input => this.inputtext = input} placeholder='message'/>
<Button onClick={this.sendMessage}>Send</Button>
</div>
);
}
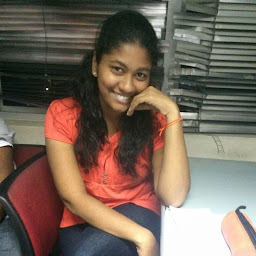
Madushika Perera
Updated on June 08, 2022Comments
-
Madushika Perera almost 2 years
I've been trying to take inputs from an
input
field and i usedrefs
(the usual way inreact
), But it doesn't seem to be working. Theinput
i'm getting isundefined
. This is my code:sendMessage = () => { console.log(this.inputtext.value); } render(){ return( <div> <Input ref={input => this.inputtext = input;} placeholder='message'/> <Button onClick={this.sendMessage}>Send</Button> </div> ); }
I need to take the inputs from the
click event
of the button. I can't figure out what's wrong. How can i get theinput
value properly? -
Madushika Perera about 7 yearsim still getting it as undefined
-
spirift about 7 yearsAs @Vikramaditya said it is due to
Input
not passing the ref prop to theinput
tag it generates. Try just using a plaininput
tag. -
Michael Ceber about 5 yearsYep this is what I do as well.