How to use multiple or two state values in one component or class file in react JS?
Solution 1
This is your state
formData:{
name: '',
address: ''
}
Change both these lines respectively
this.setState({formData:{address:value}});
this.setState({formData:{name:value}});
To
this.setState({formData:{name: this.state.formData.name, address:value}});`
this.setState({formData:{name:value, address: this.state.formData.address}});`
Solution 2
With these lines you are replacing your whole state with the value of one field:
Your initial state:
state = {
formData: {
name: "",
address: ""
}
}
Your state after this line:
this.setState({formData:{address:value}});
state = {
formData: {
address: ""
}
}
And with this line:
this.setState({formData:{name:value}});
state = {
formData: {
name: ""
}
}
As mentioned in the answer by Greg b, you have to change the value of the specific key and copy rest as they are. You can also use spread operator to copy rest of the unmodified fields easily.
this.setState({...this.state.formData, address: value})
will only change the address and copy rest of the state as it is. This comes handy when you have multiple fields in the state.
Related videos on Youtube
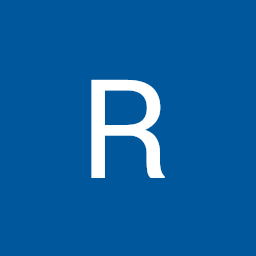
Rahul Waghmare
Updated on June 04, 2022Comments
-
Rahul Waghmare almost 2 years
I am trying to use state to hide Semantic-UI Model form also to pass JSON to Customer class to add new customer. I am able to pass the value but in the end there is only one value.
When I start typing name, in console panel on 1st character name is empty "" and on 2nd character there is single "a"
when i click create button the name is empty"" and address has value.
import React from 'react'; import { Button, Form, Modal } from 'semantic-ui-react'; export default class AddCustomer extends React.Component { constructor(props) { super(props); this.state = { showCreateForm:false, formData:{ name: '', address: '' } } this.handleChangeName = this.handleChangeName.bind(this); this.handleChangeAddress = this.handleChangeAddress.bind(this); this.handleSubmit = this.handleSubmit.bind(this); } handleChangeName(event) { const value = event.target.value; console.log(value); this.setState({formData:{name:value}}); //when i go to add input the formData is still empty name is empty. //name: "" //address: "" console.log(this.state.formData); } handleChangeAddress(event) { const value = event.target.value; console.log(value); this.setState({formData:{address:value}}); //name: "ram" but now there is no address in formData console.log(this.state.formData); } handleSubmit(event) { event.preventDefault(); ////address: "aaaaa" now there no name in formData console.log(this.state.formData); this.setState({formData:{ name:this.state.name, address:this.state.address }}); this.props.onAddFormSubmit(this.state.formData); } //On cancel button click close Create user form closeCreateForm = () => { this.setState({ showCreateForm: false }) } //Open Create new Customer form openCreateCustomer = () => { this.setState({ showCreateForm: true }) } render() { return ( <div> <Modal closeOnTriggerMouseLeave={false} trigger={ <Button color='blue' onClick={this.openCreateCustomer}> New Customer </Button> } open={this.state.showCreateForm}> <Modal.Header> Create customer </Modal.Header> <Modal.Content> <Form onSubmit={this.handleSubmit}> <Form.Field> <label>Name</label> <input type="text" placeholder ='Name' name = "name" value = {this.state.name} onChange = {this.handleChangeName}/> </Form.Field> <Form.Field> <label>Address</label> <input type="text" placeholder ='Address' name = "address" value = {this.state.address} onChange = {this.handleChangeAddress}/> </Form.Field> <br/> <Button type='submit' floated='right' color='green'>Create</Button> <Button floated='right' onClick={this.closeCreateForm} color='black'>Cancel</Button> <br/> </Form> </Modal.Content> </Modal> </div> ) } }
-
Rahul Waghmare about 4 yearsif I do like this how can i add name and address in formData