JSX map only the first object in array
18,993
Solution 1
The easiest solution would be to just not use .map
at all!
<span className="fooBar">
<a href={this.state.data[0].foo}>{this.state.data[0].bar}</a>
</span>
.map
will always go over every object in an array - there's no way to break out of it early.
Solution 2
Map is designed to call every element in an array applying a transformation.
If you just want to render the first then you'd be better to either explicitly call the first element this.state.data[0] or use a library that supports a method like head.
Lodash Example
const link = _.head(this.state.data);
<span className="fooBar">
<a href={link.href}>{link.title}</a>
</span>
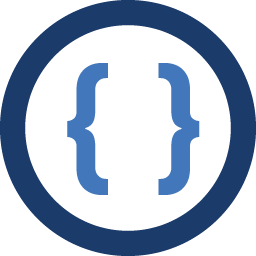
Author by
Admin
Updated on June 11, 2022Comments
-
Admin almost 2 years
I'm curious on how to only render the first object in an array using
.map
in JSX.{this.state.data.map(data => <span className="fooBar"> <a href={data.foo}>{data.bar}</a> </span> )}
Thank you!
-
budji over 5 yearsIs it possible to offset this? Like if you want the rest of the data wrapped in a different div for example.
-
Joe Clay over 5 years@budji: For the rest of the data, you could just slice the array:
this.state.data.slice(1).map(x => ...)
-
Anshuman Pattnaik about 5 yearsNot working still it's this.state.data[0] is undefined
-
Joe Clay about 5 yearsIf there is a chance that
this.state.data[0]
will be undefined, you must handle that in your code. -
Zahid Hassan Shaikot about 4 yearsI am new in reactjs. how can i use lodash in react.