Warning: Prop `className` did not match. when using styled components with semantic-ui-react
Solution 1
This warning was fixed for me by adding an .babelrc file in the project main folder, with the following content:
{
"presets": ["next/babel"],
"plugins": [["styled-components", { "ssr": true }]]
}
See following link for an example: https://github.com/nblthree/nextjs-with-material-ui-and-styled-components/blob/master/.babelrc
Solution 2
You should install the babel plugin for styled-components and enable the plugin in your .babelrc
npm install --save-dev babel-plugin-styled-components
.babelrc
{
"plugins": [
[
"babel-plugin-styled-components"
]
]
}
Solution 3
Or you could just add this to your next.config.js
. This also makes it so next-swc (speedy web compiler) works to reduce build times. See more here.
// next.config.js
module.exports = {
experimental: {
// Enables the styled-components SWC transform
styledComponents: true
}
}
Solution 4
The main reason I am posting this answer to help people understand the tradeoff. When we're using .babelrc
in next project it's going to opt of SWC compiler which is based on Rust (Learn More).
It's going to show message something like this when you opt for custom bable config.
info - Disabled SWC as replacement for Babel because of custom Babel configuration ".babelrc"
I did more digging on this to only find out following! Ref
Next.js now uses Rust-based compiler SWC to compile JavaScript/TypeScript. This new compiler is up to 17x faster than Babel when compiling individual files and up to 5x faster Fast Refresh.
So tradeoff was really huge, we can lose significant amout of performance. So I found a better solution which can solve this issue and keep SWC as default compiler.
You can add this experimental flag in your next.config.js
to prevent this issue. Ref
// next.config.js
module.exports = {
compiler: {
// ssr and displayName are configured by default
styledComponents: true,
},
}
Solution 5
If you have already added babel plugins, delete the .next build folder & restart the server again
credit: Parth909 https://github.com/vercel/next.js/issues/7322#issuecomment-912415294
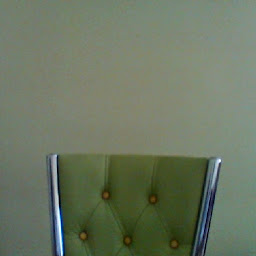
Talha Talip Açıkgöz
Updated on July 09, 2022Comments
-
Talha Talip Açıkgöz almost 2 years
I use this code to margin my Button from top:
const makeTopMargin = (elem) => { return styled(elem)` && { margin-top: 1em !important; } `; } const MarginButton = makeTopMargin(Button);
and whenever i use
MarginButton
node, I get this error:Warning: Prop
classNamedid not match. Server: "ui icon left labeled button sc-bwzfXH MjXOI" Client: "ui icon left labeled button sc-bdVaJa fKCkqX"
You can see this produced here.
What should I do?
-
brianespinosa over 5 yearsThe link you shared is to port :3000 on an IP address. Unless you have made that IP address available to public traffic and have a currently running web server there, nobody will be able to access what you are running. Try creating a minimal example of your problem using a tool like codesandbox instead. codesandbox.io
-
Talha Talip Açıkgöz over 5 yearsSorry, that ip is actually a vps but it is not running right now for a reason. I will try to reproduce it now.
-
Talha Talip Açıkgöz over 5 years@brianespinosa Please see codesandbox.io/s/xvmq9jjzzq
-
brianespinosa over 5 yearsI am looking at your console and there is no proptype warning for className.
-
Talha Talip Açıkgöz over 5 yearsI think it happens only in development environment. I can't reproduce it online.
-
simpleProgrammer almost 3 yearsI have this error happening two pages, none of the answers helped, did you end resolving this ?
-
VeRJiL about 2 yearsYou only need to add this line of code into your next.config.js file
module.exports = { compiler: { styledComponents: true, } }
-
-
nwillo almost 4 yearsI have tried to add styled-components to my Next.js project. It still doesn't work. My issue is that when I reload the page deployed by npm run dev, it throws the error of server not matching the styles at the client end. I have even used the babel-plugin-styled-component plugin. Nothing seems to be working. Can you suggest something? Thanks!
-
Dan about 3 yearsThis worked for me after I
npm i babel-preset-next
-
VRedondo over 2 yearsSo this works for me, but what's the explanation for this ?
-
Dani Amsalem over 2 yearsThis worked well for me. All I had to do afterwards was restart my local server. Based on my limited understanding, it seems that this experimental value prevents mismatched classes created by styled-components as it is processed by the server and then the client.
-
Jason Frank about 2 yearsAs of Feb 2022 it appears that this no longer "experimental". As @Abhilash Rathod points out in his answer (stackoverflow.com/a/71100071/718325), it now works under the
compiler
key instead ofexperimental
. -
Farid shahidi about 2 yearsAfter install
npm i babel-preset-next
I couldn't start my project :( -
cantaş about 2 yearsi used babel-plugin-styled-components package and RESTART project. it works