Increment and Decrement operators in scheme programming language
Solution 1
They are not defined as such since Scheme and Racket try to avoid mutation; but you can easily define them yourself:
(define-syntax incf
(syntax-rules ()
((_ x) (begin (set! x (+ x 1)) x))
((_ x n) (begin (set! x (+ x n)) x))))
(define-syntax decf
(syntax-rules ()
((_ x) (incf x -1))
((_ x n) (incf x (- n)))))
then
> (define v 0)
> (incf v)
1
> v
1
> (decf v 2)
-1
> v
-1
Note that these are syntactic extensions (a.k.a. macros) rather than plain procedures because Scheme does not pass parameters by reference.
Solution 2
Your reference to “DrRacket” somewhat suggests you’re in Racket. According to this, you may already be effectively using #lang racket
. Either way, you’re probably looking for add1
and sub1
.
-> (add1 3)
4
-> (sub1 3)
2
Solution 3
The operators 1+ and -1+ do /not/ mutate, as a simple experiment in MIT Scheme will show:
1 ]=> (define a 3)
;Value: a
1 ]=> (1+ a)
;Value: 4
1 ]=> (-1+ a)
;Value: 2
1 ]=> a
;Value: 3
So you can implement your own function or syntactic extensions of those functions by having them evaluate to (+ arg 1) and (- arg 1) respectively.
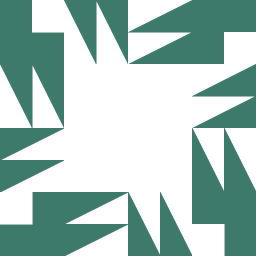
unknownerror
Updated on June 14, 2022Comments
-
unknownerror 3 months
What are the
increment
anddecrement
operators inscheme
programming language. I am using "Dr.Racket" and it is not accepting-1+
and1+
as operators. And, I have also triedincf
anddecf
, but no use. -
unknownerror over 5 yearsThis will not change that value of variable you are passing. Have a look here: ideone.com/KEgO9g