File I/O operations - scheme
Solution 1
It's mostly implementation-specific. Given that you're using racket, see the guide section and the reference manual.
Solution 2
The easiest way to read/write files in any R5RS compliant Scheme is:
;; Read a text file
(call-with-input-file "a.txt"
(lambda (input-port)
(let loop ((x (read-char input-port)))
(if (not (eof-object? x))
(begin
(display x)
(loop (read-char input-port)))))))
;; Write to a text file
(call-with-output-file "b.txt"
(lambda (output-port)
(display "hello, world" output-port))) ;; or (write "hello, world" output-port)
Scheme has this notion of ports that represent devices on which I/O operations could be performed. Most implementations of Scheme associate call-with-input-file
and call-with-output-file
with literal disk files and you can safely use them.
Solution 3
Please see the following post if you are working with a R5RS compliant Scheme:
R5RS Scheme input-output: How to write/append text to an output file?
The solution presented there is as follows:
; This call opens a file in the append mode (it will create a file if it doesn't exist)
(define my-file (open-file "my-file-name.txt" "a"))
; You save text to a variable
(define my-text-var1 "This is some text I want in a file")
(define my-text-var2 "This is some more text I want in a file")
; You can output these variables or just text to the file above specified
; You use string-append to tie that text and a new line character together.
(display (string-append my-text-var1 "\r\n" my-file))
(display (string-append my-text-var2 "\r\n" my-file))
(display (string-append "This is some other text I want in the file" "\r\n" my-file))
; Be sure to close the file, or your file will not be updated.
(close-output-port my-file)
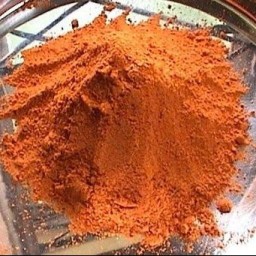
JJunior
Updated on June 04, 2022Comments
-
JJunior 6 months
Can someone point me to basic file I/O operations examples in Scheme?
I just want to try basic read/write/update operations on a file.
Finding it difficult as not having appropriate resources to learn from.