iOS 11 UISearchBar in UINavigationBar
Solution 1
Now it's what you want...
if #available(iOS 11.0, *) {
let sc = UISearchController(searchResultsController: nil)
sc.delegate = self
let scb = sc.searchBar
scb.tintColor = UIColor.white
scb.barTintColor = UIColor.white
if let textfield = scb.value(forKey: "searchField") as? UITextField {
textfield.textColor = UIColor.blue
if let backgroundview = textfield.subviews.first {
// Background color
backgroundview.backgroundColor = UIColor.white
// Rounded corner
backgroundview.layer.cornerRadius = 10;
backgroundview.clipsToBounds = true;
}
}
if let navigationbar = self.navigationController?.navigationBar {
navigationbar.barTintColor = UIColor.blue
}
navigationItem.searchController = sc
navigationItem.hidesSearchBarWhenScrolling = false
}
Result:
With Rounded corner:
Animation with rounded corner is also working fine.
Solution 2
I changed the background of the text field with this code inside AppDelegate.
Swift 4
func application(_ application: UIApplication, didFinishLaunchingWithOptions launchOptions: [UIApplicationLaunchOptionsKey: Any]?) -> Bool {
//background color of text field
UITextField.appearance(whenContainedInInstancesOf: [UISearchBar.self]).backgroundColor = .cyan
}
This is the result
Solution 3
Objective C
if (@available(iOS 11.0, *)) {
self.searchController = [[UISearchController alloc] initWithSearchResultsController:nil];
self.searchController.searchResultsUpdater = self;
self.searchController.searchBar.delegate = self;
self.searchController.dimsBackgroundDuringPresentation = NO;
self.navigationItem.searchController=self.searchController;
self.navigationItem.hidesSearchBarWhenScrolling=NO;
self.searchController.searchBar.searchBarStyle = UISearchBarStyleProminent;
self.searchController.searchBar.showsBookmarkButton = NO;
self.searchController.searchBar.placeholder = @"Search";
self.searchController.searchBar.tintColor = [UIColor colorWithRed:1 green:1 blue:1 alpha:1];
self.searchController.searchBar.barTintColor=[UIColor colorWithRed:1 green:1 blue:1 alpha:1];
UITextField *txfSearchField = [self.searchController.searchBar valueForKey:@"_searchField"];
txfSearchField.tintColor=[UIColor colorWithRed:21/255.0 green:157/255.0 blue:130/255.0 alpha:1];
txfSearchField.textColor=[UIColor colorWithRed:1 green:1 blue:1 alpha:1];
txfSearchField.backgroundColor=[UIColor whiteColor];
UIView *backgroundview= [[txfSearchField subviews]firstObject ];
backgroundview.backgroundColor=[UIColor whiteColor];
// Rounded corner
backgroundview.layer.cornerRadius = 8;
backgroundview.clipsToBounds = true;
}
Solution 4
This should work for you
func addSearchbar(){
if #available(iOS 11.0, *) {
let sc = UISearchController(searchResultsController: nil)
let scb = sc.searchBar
scb.tintColor = UIColor.white
if let navigationbar = self.navigationController?.navigationBar {
//navigationbar.tintColor = UIColor.green
//navigationbar.backgroundColor = UIColor.yellow
navigationbar.barTintColor = UIColor.blue
}
navigationController?.navigationBar.tintColor = UIColor.green
navigationItem.searchController = sc
navigationItem.hidesSearchBarWhenScrolling = false
}
}
Result:
Solution 5
This is the code I used to make the search bar white:
if let textfield = searchController.searchBar.value(forKey: "searchField") as? UITextField {
if let backgroundview = textfield.subviews.first {
backgroundview.backgroundColor = UIColor.init(white: 1, alpha: 1)
backgroundview.layer.cornerRadius = 10
backgroundview.clipsToBounds = true
}
}
Related videos on Youtube
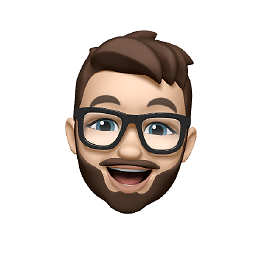
Darko
Just loving Apples "no-compromise-on-quality" philosophy and their great products.
Updated on October 05, 2022Comments
-
Darko less than a minute
I want to place a search bar in the new navigation bar with the new iOS 11 large titles. However, the color of the search bar is automatically applied by iOS and I can't change it.
if #available(iOS 11.0, *) { let sc = UISearchController(searchResultsController: nil) navigationItem.searchController = sc navigationItem.hidesSearchBarWhenScrolling = false }
The search bar get's a deep blue background, but I just want to change it to white.
Setting the background color results in this:
navigationItem.searchController?.searchBar.backgroundColor = UIColor.white
I have tried also
setScopeBarButtonBackgroundImage
andsetBackgroundImage
on the search bar but everything looks totally weird.Also when I trigger the search by tapping on the search bar, it switches to a mode with the cancel button on the right side. ("Abbrechen" in German)
And the "Abbrechen" text color also can't be changed. (need it also white)
Any help is appreciated.
Edit: As requested, here the code for the navigation bar styling:
self.navigationBar.tintColor = UIColor.myWhite self.navigationBar.titleTextAttributes = [NSAttributedStringKey.foregroundColor : UIColor.myWhite, NSAttributedStringKey.font: UIFont.myNavigationBarTitle()] self.navigationBar.barTintColor = UIColor.myTint if #available(iOS 11.0, *) { self.navigationBar.prefersLargeTitles = true self.navigationBar.largeTitleTextAttributes = [NSAttributedStringKey.foregroundColor : UIColor.myWhite, NSAttributedStringKey.font: UIFont.myNavigationBarLargeTitle()] }
Current outcome: I have used Krunals idea to set the color of the search bar background but then the rounded corners are lost. After re-setting the rounded corners the animation of the search bar seems to be broken.
So still no satisfactory solution. Seems that the search bar when embedded into the navigation bar in iOS 11 is not possible to customize. Meanwhile it would be enough for me to just change the color of the placeholder text, but even this seems not to be possible. (I have tried multiple approaches from StackOverflow - doesn't work)
-
Darko about 5 years
if let textField = navigationItem.searchController?.searchBar.value(forKey: "_searchField") as? UITextField { textField.backgroundColor = UIColor.white }
- this does not work. -
Darko about 5 yearsThis is exactly what I already have. I need the search bar to have a white background.
-
Darko about 5 yearsIt really seems that this is actually not possible in iOS 11.
-
Darko about 5 yearsYes, thirst success, thanks! The rounded edges would also be possible over view.layer I suppose. Accomplish this also and I will mark it as answered, for others to find this. The inside knowledge about the inner workings is also not the most preferable way to achieve it, but if that's the only way...
-
Toby Speight almost 5 yearsWelcome to Stack Overflow! Thank you for this code snippet, which might provide some limited, immediate help. A proper explanation would greatly improve its long-term value by showing why this is a good solution to the problem, and would make it more useful to future readers with other, similar questions. Please edit your answer to add some explanation, including the assumptions you've made.
-
Helge Becker almost 4 yearsHow did you get the searchbar below the large title?
-
iOS_Mouse almost 4 yearsThe searchbar is placed below the large title by default if you add a search controller to the navigation bar. In code I created a search controller in the ViewDidLoad-- let controller = UISearchController(searchResultsController: nil) -- followed by setting up all the features for "controller". Then I added the search controller to the navigation -- navigationItem.searchController = controller -- followed by some more setup
-
Jonathan Cabrera almost 4 yearsThat's not white, it's a very light gray
-
Satsuki over 2 yearsHi I have tested this code and it works great on iOS 13 devices but seems to have problems in iOS 12. Is this the case with anyone?