Is there a way to automatically generate getters and setters if they aren't present in C++?
Solution 1
The C++ Core Guidelines advise against using trivial getters and setters because they’re unnecessary and a symptom of bad object-oriented design. As such, C++ has no built-in functionality for auto-generating getters and setters (though metaclasses, if they ever get included in the language, would make this possible). This is related to the well-established software engineering principle tell, don’t ask.
In particular, mutating state via setters is usually a sign of code smell and a bad architectural design. There are exceptions from this rule, purely out of practicality. And this is fine, but the exceptions are few enough that they shouldn’t warrant tools to auto-generate getters and setters.
In fact, you may use this as a litmus test: whenever you find yourself wishing for a tool to autogenerate such boilerplate, take a step back and reconsider your code design.
That said, there exist a number of tools to provide the functionality and in purely practical terms they may prove useful, though I have not personally tested them:
- Visual Studio Code:
- Vim
- Emacs
- Visual Studio
-
CLion
- built in
-
Eclipse
- built into Exclipse CDT (“Implement method”)
Solution 2
Not the compiler itself, but an IDE like eclipse CDT can actually perform this action automatcally (right click on class > Source > Generate Getters and Setters...).
Solution 3
You have to implement them yourself
Solution 4
There is no way to do this. However, if you use a fully-featured IDE like Eclipse (not sure if Visual Studio has this feature), there are convenience options to have the IDE generate the getter/setter code for you.
Solution 5
The advantage for me of using accessors (specialy easy in the form of c# vb.net) is
1/ the possibility to set a break point in a setter or a getter else you cannot know who and when has access to the member.
2/ You can control what value is set in case of need to test overflow or other bad set. This is specially needed in a public libray, like a property editor for your visual component by example.
But this is not fully needed in your private internal class. Then the choice of a controlled access to a member must be set from the final public usage of your object I think.
Related videos on Youtube
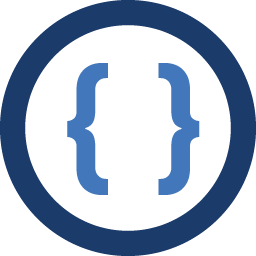
Admin
Updated on November 28, 2020Comments
-
Admin over 3 years
I'm experienced with Objective-C, and in Objective-C you can let the compiler generate getters and setters for you if they aren't already present (
@synthesize
).Is there a way to do this in C++, or do I need to implement all getters and setters myself?
-
Seif Sarsar almost 5 yearsThere is an extension in Visual Studio 2017 called GS Assist. It gives you the possibility to create getters and setters quickly in C++. Here is the link : marketplace.visualstudio.com/items?itemName=SeifSarsar.gsassist
-
snips-n-snails over 3 yearsWhat I'd really like are implicit getters and setters, to avoid cluttering my class that uses the Named Parameter Idiom.
-
-
flumpb almost 13 yearsCould you recommend me a modern c++ guideline article/book?
-
Eugene S almost 13 yearsIf you are using Eclipse CDT, you can find instructions on how to generate getters/setters here.
-
stijn almost 13 yearsisn't this a tad (or even more) too general? Eg this statement doesn't make much sense for containers.
-
Konrad Rudolph almost 13 years@stijn It’s a general guideline. As such, it’s general but that doesn’t mean it can’t be broken occasionally. And it actually makes a great deal of sense for containers. How many containers in the C++ standard library have setters? Right, zero. How many getters have they got? Very few: essentially
size
,empty
and perhapscapacity
. -
stijn almost 13 yearsah I guess I misunderstood the whole 'setters/getters' then. I was under the impression that operator [] would be considered an accessor/getter..
-
KillianDS almost 13 years@Konrad: is usage of getters in an ORM system an exception or is ORM wrong in itself (likewise for MVC)? same q, otherwise stated: are those patterns themselves correct and justify exception to this rule or are they in fact 'ugly' and an inheritance of getter/setter abuse?
-
C.J. almost 13 yearsMacro's = difficult or impossible to debug
-
duedl0r almost 13 yearsyawn...is this your auto response if somewhere the word MACRO is used?
-
Konrad Rudolph almost 13 years@KillianDS ORM is arguably wrong – other people have argued that ORMs all eventually break down in real projects (can’t find a link now, sorry). But they work well enough in most small and perhaps even medium sized projects, so why chuck them out? I’d therefore say that ORMs are an exception. That said, ORMs can easily be designed to have immutable objects that work with builders, so even ORMs can be designed setter-free without problem. I’ve already used such a (handwritten) ORM, it worked great. As for MVC, that’s actually addressed in the comments of the linked article.
-
David Stone about 12 years
empty
isn't even really a plain getter, in the sense that it's not comprised ofreturn member_variable;
. -
David Stone about 12 yearsIf you give every variable a
get
andset
function, that's not really that different from just making your variables public. -
Konrad Rudolph about 12 years@David In most definitions it’s still a getter. Getters and setters can implement logic – that’s the whole point of them; otherwise it would make no sense to use them instead of public variables.
-
David Stone about 12 yearsWhen I hear the term, I think of a getter as a function that simply returns a member variable, and a setter as something that takes a value as input and sets the variable to that value, with possible additional checking added in.
-
Ramy Al Zuhouri over 11 yearsHow to set the rgb color for a "color" class then?
-
Konrad Rudolph over 11 years@RamyAlZuhouri By creating a new instance obviously.
-
Ramy Al Zuhouri over 11 yearsBut for mutable classes I need a setter if I want to mutate the color.
-
Konrad Rudolph over 11 years@Ramy Sure but how often is that actually happening? We already mentioned one case above: ORM. But that’s the exception. Often you don’t actually need to change an object’s properties after creating it, or when you do, it makes more sense to re-create the object from scratch (after all, that’s what’s happening with strings in .NET!)
-
Admin over 11 years@RamyAlZuhouri you make the color public problem solved. In fact, a color class needs to be nothing more than
struct color { double red, green, blue; };
. Everything else can be done through free functions (preferably pure ones). -
Ramy Al Zuhouri over 11 yearsMaking color public means that the class is still mutable, it's like having a getter/setter, with the difference that you can't control the code executed when calling a setter, thus you have less control. I don't believe that is wise to program completely without setters/getters, it's close to the impossible, and also very inefficient and unwise.
-
Konrad Rudolph over 11 years@Ramy I can assure you that it’s absolutely possible to do that, I’ve done it, it works fine in some (most, I’d argue) situations. In fact, functional programming languages do that all the time and nobody is complaining. (And no, not by using public variables either.)
-
Ramy Al Zuhouri over 11 yearsI don't believe it, everywhere you need to use a sort of setter, unless you find some trick to don't use them, but using something of equivalent. A program is made of memory and instructions, if you only use immutable objects your program's state will never change, thus you can't make any choice at runtime.
-
Ramy Al Zuhouri over 11 yearsAlso I down voted it, because you should have said when to use immutable objects, and when you shouldn't use them, there are different cases, you shouldn't say to don't use immutable objects at all, if so what is our good sense made for? Should we always choose things so blindly? Your second point says: "You create a new instance.". Well in this exact case, even if the object itself doesn't change, the pointer to it changes, so you need to do it in a mutable class. You always need something mutable in a program.
-
Ramy Al Zuhouri over 11 yearsAnd sorry if I type so much, but I would also say that we as programmers, are made to make choices and to decide when to use a pattern instead of another, and there is always a reason for this. We don't just have to always follow the same pattern, saying it's "good" vs the "evil" one, otherwise we wouldn't be programmers at all.
-
Konrad Rudolph over 11 years@Ramy Ah this is really a quite boring debate, especially for comments. This is textbook knowledge. If you’re interested in the debate, learn about functional programming and learn a functional programming language (I suggest Haskell). Then come back. Before you’ve got this factual basis a discussion really makes no sense.
-
Ramy Al Zuhouri over 11 yearsWe are talking about C++ so I don't have to study Haskell for it. C++ 's strong point isn't functional programming.
-
Konrad Rudolph over 11 years@Ramy That’s quite irrelevant. You are arguing against a wealth of research and practical knowledge. For this discussion to be productive you need to catch up on this knowledge, and the best way of doing this is by learning functional programming. That doesn’t mean that the facts don’t apply to C++. Quite the contrary: object oriented folks have long since discovered this truth for themselves.
-
Sava B. over 8 yearsI hate this answer. I don't disagree with "Having as few setters/getters as possible is best". But sometimes it makes sense. Even the author of article in the answer says: "I suggested avoiding accessors as much as is practical. You will run into cases where accessors are cleaner than any other solution...". Suppose I need to check the velocity of an object in some gameplay code. Gameplay code doesn't belong in the object. What I need is a getter for the velocity. Immutable objects are great, but not performant. OP asked how to do something. Tell them how. Don't question their motives.
-
Konrad Rudolph over 8 years@SavaB. OP seems to be happy with the answer, as in: it seems to have solved their underlying problem. And regarding your gaming example, there are ways of making this work, though they require fundamentally different API decisions. But, as mentioned in other comments, I'm not dead set against setters. What I'm dead set against is such an abundance of setters that there's need to autogenerate them. That is most certainly code smell.
-
Sava B. over 8 yearsSomeone might be looking for a way to generate them because it's faster than writing them by hand, whether there are many or few. These questions are really for everyone, not just OP (for example, I stumbled upon this question). I think a direct answer to the question with an aside about poor design would have been much better. Also, my example was very contrived, but my point is that projects have different sizes, requirements, legacy code, coding styles... People throw around "You'd never do that", but it's rare that you can say this about the universe of all possible programming work.
-
Konrad Rudolph over 8 years@SavaB. Well like I’ve said, I believe that you shouldn’t have many setters, and that, when you do, the solution is to rethink your API, not find a generator to soothe the symptoms of the code smell. I’m not interested in providing quick&dirty solutions here, I’m interested in helping people write better code. That said, nothing prevents you from providing your own answer. In fact, my answer explicitly says that it isn’t the answer-for-all. It’s hardly my fault that OP decided that this solved their problem, and accepted it. It was meant to stand alongside other answers.
-
magallanes almost 8 yearsCode smells is to have a mix of "ideologies" mixed. If you use setter and getter then fine. If not then fine too. However, if you use both then, you are calling for troubles. Also, depending the compiler, some of them are smart and they recognize the setter and getter and could optimizes the code. What i learned is that less lines doesn't specifically means a better code. My 2cents.
-
user2164703 over 5 yearsDid your answer was relative to public getters/setters ? What if I left these getters/setters private, they could centralized access to variable so its easier to debug or upgrade the code ?
-
Makogan over 4 yearsI vehemtnly disagree with the idea that getters and setters are bad. Most of the time I need my objects to just hold a highly mutable state e.g a camera object. The reason why I don;t make the fields public is because putting a breakpoint in the setter is easier for debugging. Don;t tell people "x is a bad idea" there always exists a problem domain where what you say is wrong, be it embedded, real time, scientific computation...
-
Konrad Rudolph over 4 years@Makogan The whole point of software engineering is to determine whether some programming practices are bad ideas. That’s how we progress. Anyway, I don’t see what your comment adds, since the caveats are already mentioned in my answer. Note also that my answer does not advise making fields public, and if this is your take-away from the answer you fundamentally haven’t understood it.
-
facetus over 3 yearsHaving getters/setters greately helps debugging and testing, and can save weeks of hard work. No getters/setters is fine only with really trivial types like
Point
in the Core Guidelines. In all other cases a public data member can lead to all sorts of trouble. If it is accessed from one hundred places and you want to find from where it was set to 42 and then to 24, it will be your bad day. Hardware breakpoints can help, but sometimes it can be very cumbersome. I once had to write a fake int type and recompile to debug access to a public field. After that experience I always use get/set. -
Konrad Rudolph over 3 years@facetus You’ve fundamentally misunderstood the answer. It isn’t advising the use of public data members. Please read all the linked articles, not just the C++ core guideline.