Is there any way to check if source is subscribed?
Solution 1
It seems you can check whether the subscription is closed with
this.subscription.closed
indicate whether this Subscription has already been unsubscribed.
Solution 2
I had a similar case, I had a if condition with one optional subscribe:
if (languageIsSet) {
// do things...
} else {
this.langSub = this.langService.subscribe(lang => {
// do things...
});
}
If you want to call unsubscribe safely (you don't know if is subscribed or not), just initialize the subscription with EMPTY instance:
private langSub: Subscription = Subscription.EMPTY;
Now you can unsubscribe without errors.
Solution 3
You can check if Subject
has observers because it has a public property observers
.
With Observable
s you can't because they don't typically have arrays of observers. Only if you've multicasted them via a Subject
with the multicast()
operator for example.
Maybe if you could describe your use case in more detail I'll be able to give you better advice.
const source = ...;
let subscribed = false;
Rx.Observable.defer(() => {
subscribed = true;
return source.finally(() => { subscribed = false });
})
Solution 4
As I can see in your code you always create a subscription.
So if you created subscriptions object it means subscription exists and you can unsubscribe.
It Still a bit not clear why you need to check is any subsection exist
Btw. unsubscribe() method checking is subscription closed or not. Subscription is closed if somebody called unsubscribe() or observable is compleated
Related videos on Youtube
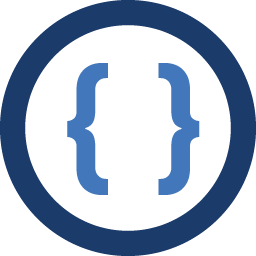
Admin
Updated on June 30, 2022Comments
-
Admin almost 2 years
Just as the title says, in Angular 2, is there any way to check if source is already subscribed? Because I have to check it before using
this.subscription.unsubscribe();
This is my code:
this.Source = Rx.Observable.timer(startTime, 60000).timeInterval().pluck('interval'); this.Subscription = this.Source .subscribe(data => { something }
and then I want to be sure that it is subscribed before calling
unsubscribe()
-
martin over 6 yearsYou want to check if Observable has observers?
-
Sergey Karavaev over 6 yearsIt is still unclear why exactly you want to check for subscription before calling
unsubscribe
. You can always initialize your subscription with an empty subscription instance, e.g.this.subscription = Subscription.EMPTY
, callingunsubscribe
on it is safe.
-
-
Max Koretskyi over 6 yearsOnly if you've multicasted - but that in effect still returns a subject, correct?